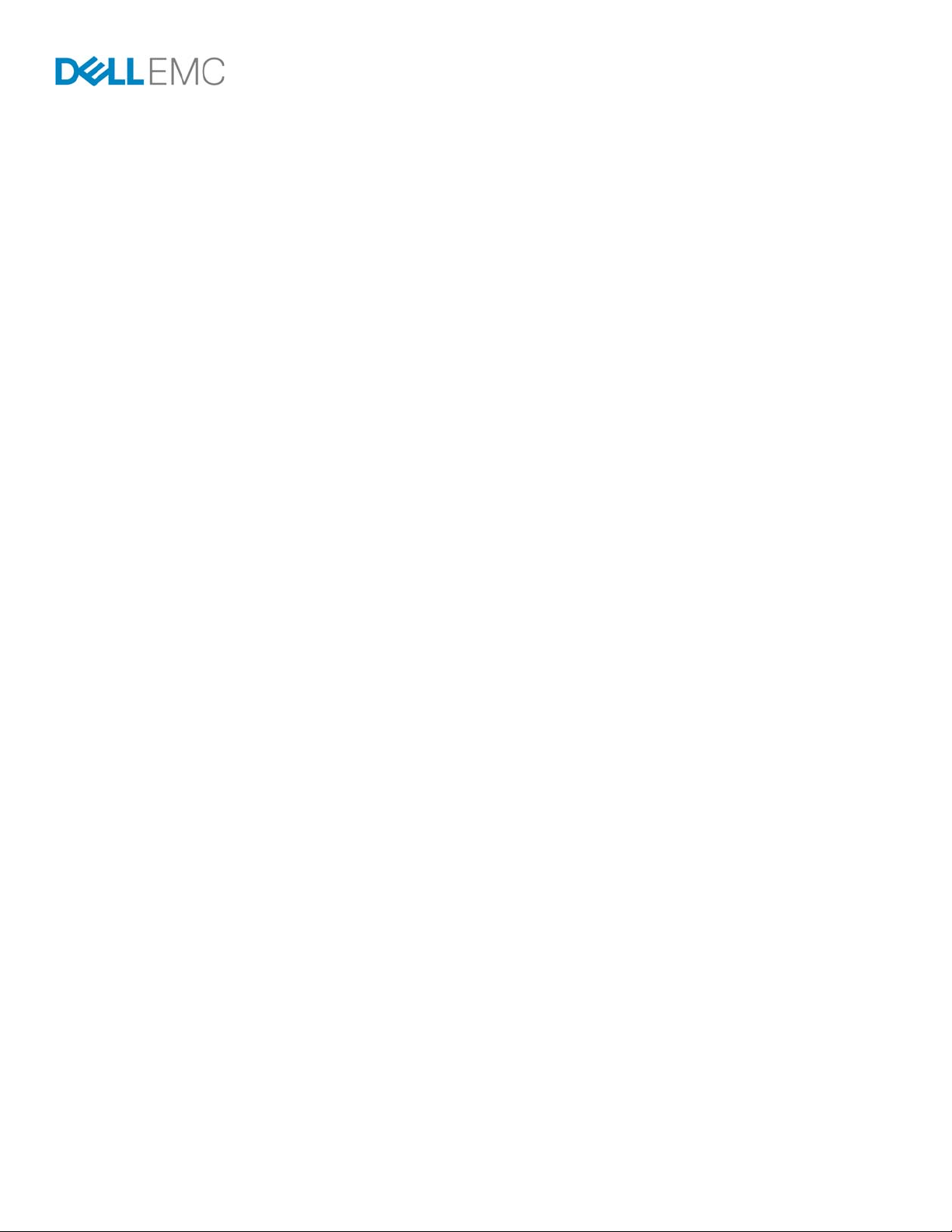
CONTROL THE GPIO PINS ON THE DELL
EMBEDDED BOX PC 5000 IN UBUNTU LINUX
ABSTRACT
The Dell Embedded Box PC 5000 provides General Purpose I/O (G PIO ) pins for
customization. Due to the unavailability of the corresponding kernel driver, users who
run the Linux system on the Box PC face a problem in controlling the GPIO pins. T his
white paper describes how to control the GPIO pins by reading/writing IO ports in a
user-space program. The audience of this document should have programming
knowledge in the C programming language on Linux oper ating systems.
March, 2017
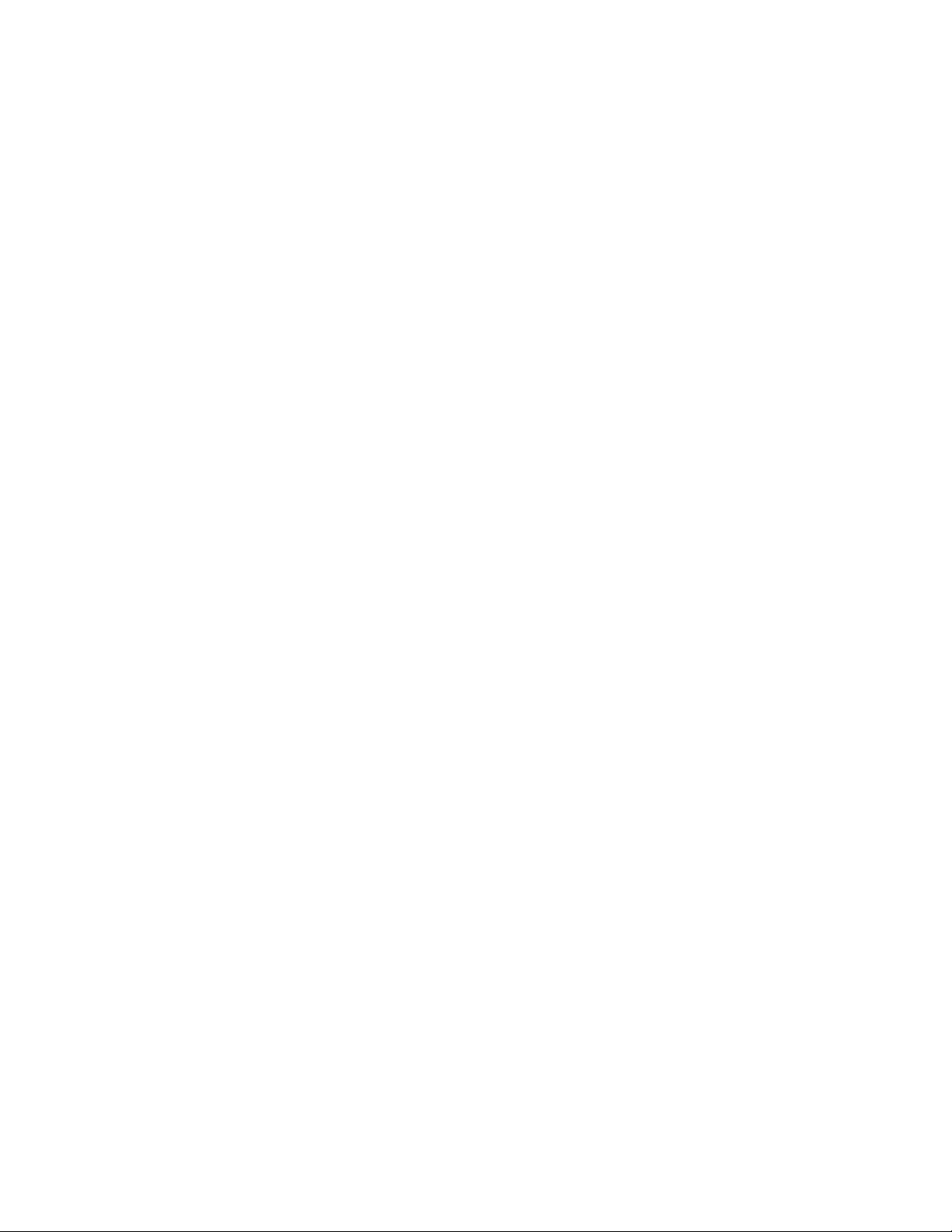
TABLE OF CONTENTS
GPIO ON DELL EMBEDDED BOX PC 5000 ............................................................................3
RESERVE THE IO RANGE FOR CALLER PROCESS ............................................................3
ACCESS THE SUPER IO CHIP ................................................................................................4
ACCESS THE GPO (OUTPUT) PINS ........................................................................................5
READ THE GPI (INPUT) PINS ..................................................................................................6
APPENDIX: FULL SOURCE CODE OF AN EXAMPLE PROGRAM .......................................7
2
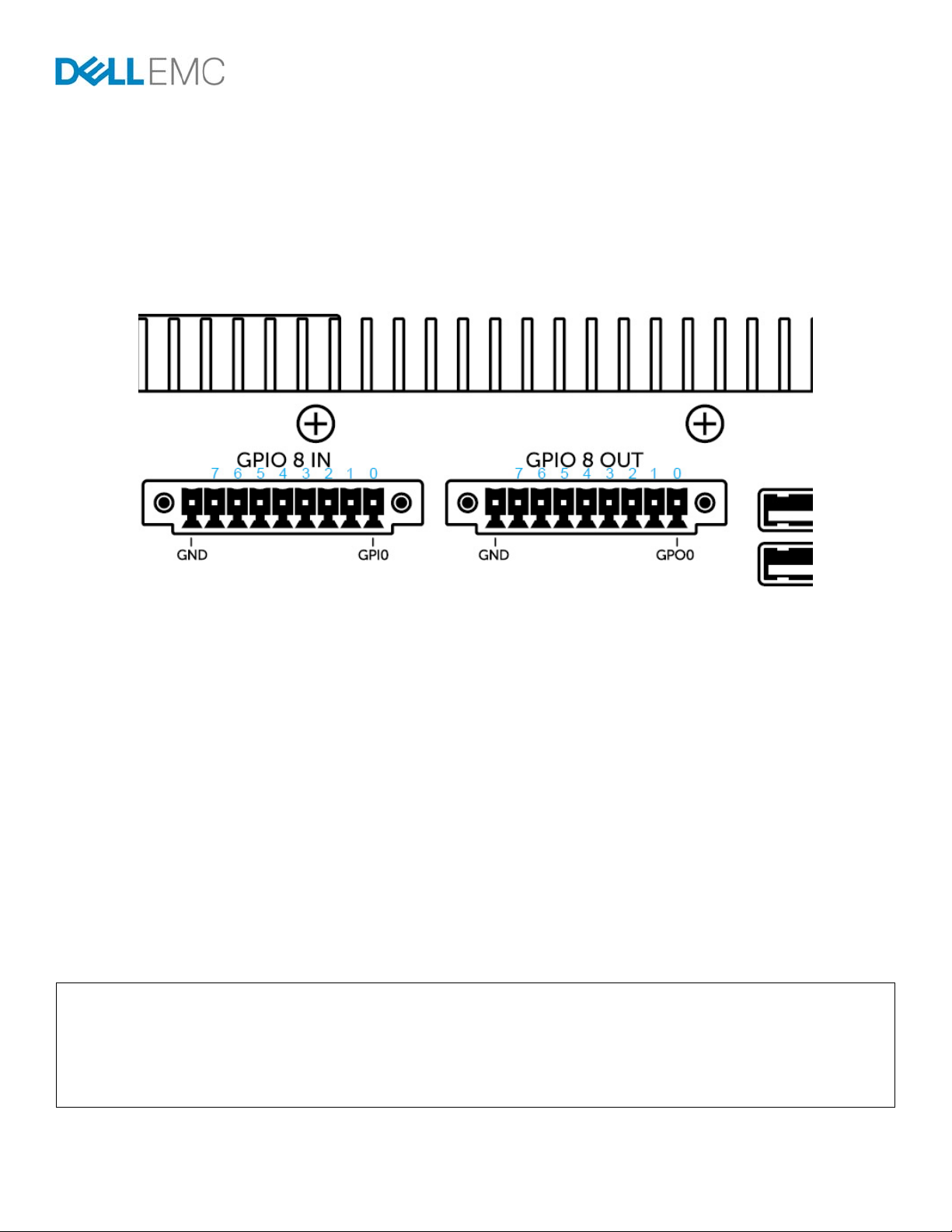
GPIO ON DELL EMBEDDED BOX PC 5000
The Dell Embedded Box PC 5000 provides 16 General Purpose I/O (G PIO ) pins organized into two groups of eight pins:
GPIO 8 IN (GPI0 to GPI7) for input
GPIO 8 OUT (GPO0 to GPO7) for output.
The pins are located on the front panels of the Embedded Box PC 5000. See Figure 1 for the placements.
Figure 1
The GPIO pins are implemented by an on-board Super IO chip, the Nuvoton NCT6793D. The controller interfaces with the host
processor through the Low Pin Count (LPC) bus.
Depending on the hardware design of the Intel Architecture-based PC, the soft ware accesses the LPC d evice b y readi ng or writing to
the 2Eh/2Fh or 4Eh/4Fh IO ports.
On the Embedded Box PC 5000, the Super IO chip is accessed through the 2Fh/2Fh IO ports. The Super IO is typicall y controlled by
the kernel driver or by the BIOS. This document describes an alternative approach to control the Super IO in a user space program
which reads and writes the IO ports.
RESERVE THE IO RANGE FOR CALLER PROCESS
Before the user-space program can access any ports, it has to gain the permission to do so. This is done by calling th e ioperm function
before any I/O port can be accessed. The call of ioperm requires the program to have root privileges – you can either run the program
as the root user or make it setuid root.
See the source code example shown below. It gives access to two IO ports 0x2E through 0x2F. The last argument is a Boolean value
specifying whether to grant or to remove the access.
#include <unistd.h>
...
ioperm(0x2E, 2, 1);
setuid(getuid()); // to drop root privileges, if this is a setuid-root file
3
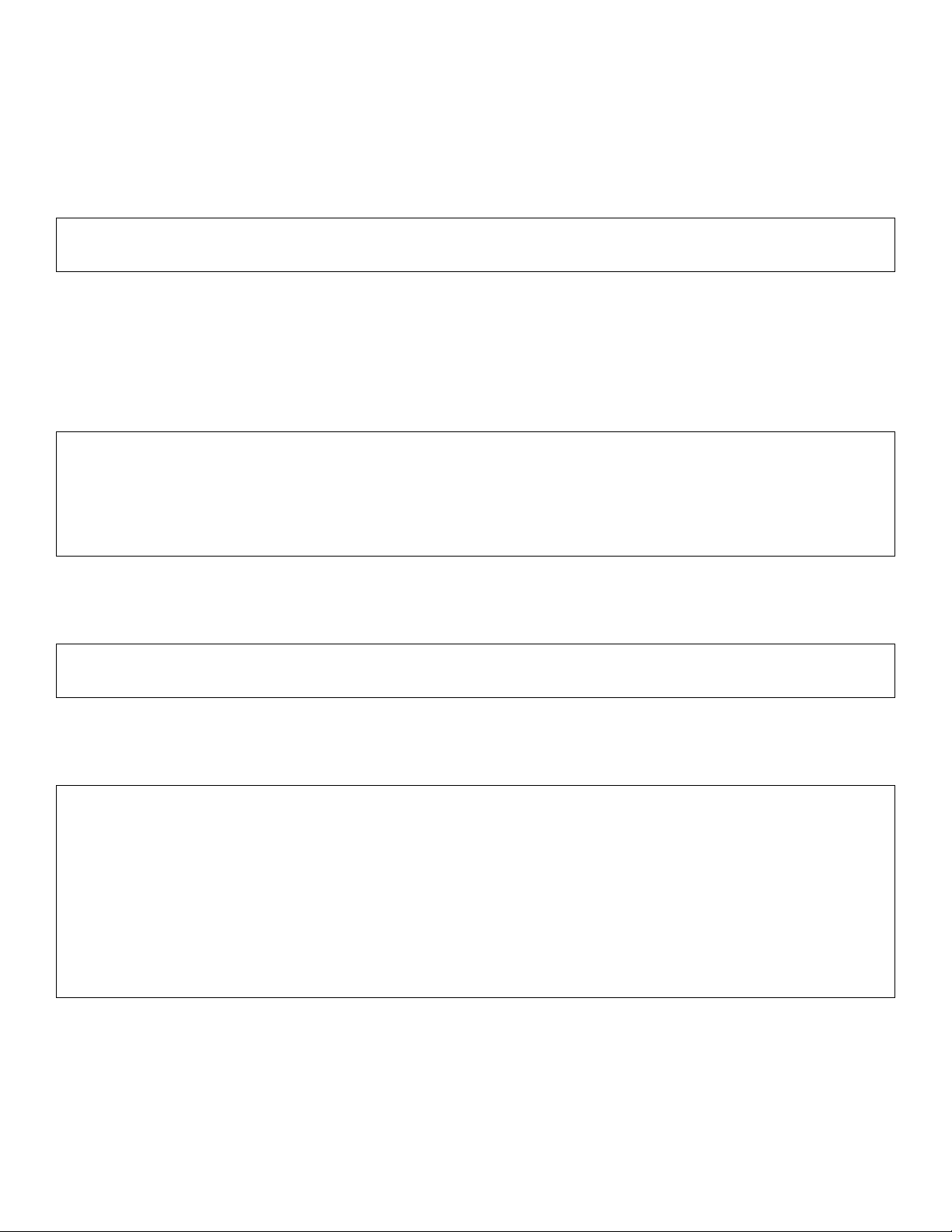
The root privileges can be dropped after calling the ioperm function. A setuid() to a non-ro ot user does not disable the access to the
ports 2Eh and 2Fh, whose permissions have been granted in the example source code.
The permission to access the IO port can be dropped at the end of the program by using the source code shown below. This is optional
because the permission is removed with the process as it exits.
ioperm(0x2E, 2, 0);
ACCESS THE SUPER IO CHIP
The Super IO chip supports multiple functions such as monitoring fan speeds and power supply voltage, providing I2C bus interface
and UART ports. GPIO is one the functionalities provided by the chip.
All accesses to the Super IO are made through the IO ports; 2Eh and 2Fh. The read/write operation performed on these ports are
transformed into LPC bus cycles for the host processor to interact with the Super IO.
#define LPC_ADDR_PORT 0x2E
#define LPC_DATA_PORT 0x2F
...
outb(0x87, LPC_ADDR_PORT);
outb(0x87, LPC_ADDR_PORT);
In the above sample source code, the byte 0x87 is written twice in a row to the IO port 0x2E. This allows the Super IO chip to enter its
Extended Function Mode, where data updates to the chip are allowed.
To leave this mode and for safety reasons, write 0xAA to the same port as shown below:
outb(0xAA, LPC_ADDR_PORT);
As the access and exit of the Extended Function Mode are frequently needed in the program, rewrite them into function s for ease of
use:
void enter_extended_function_mode()
{
outb(0x87, LPC_ADDR_PORT);
outb(0x87, LPC_ADDR_PORT);
}
void leave_extended_function_mode()
{
outb(0xAA, LPC_ADDR_PORT);
}
4