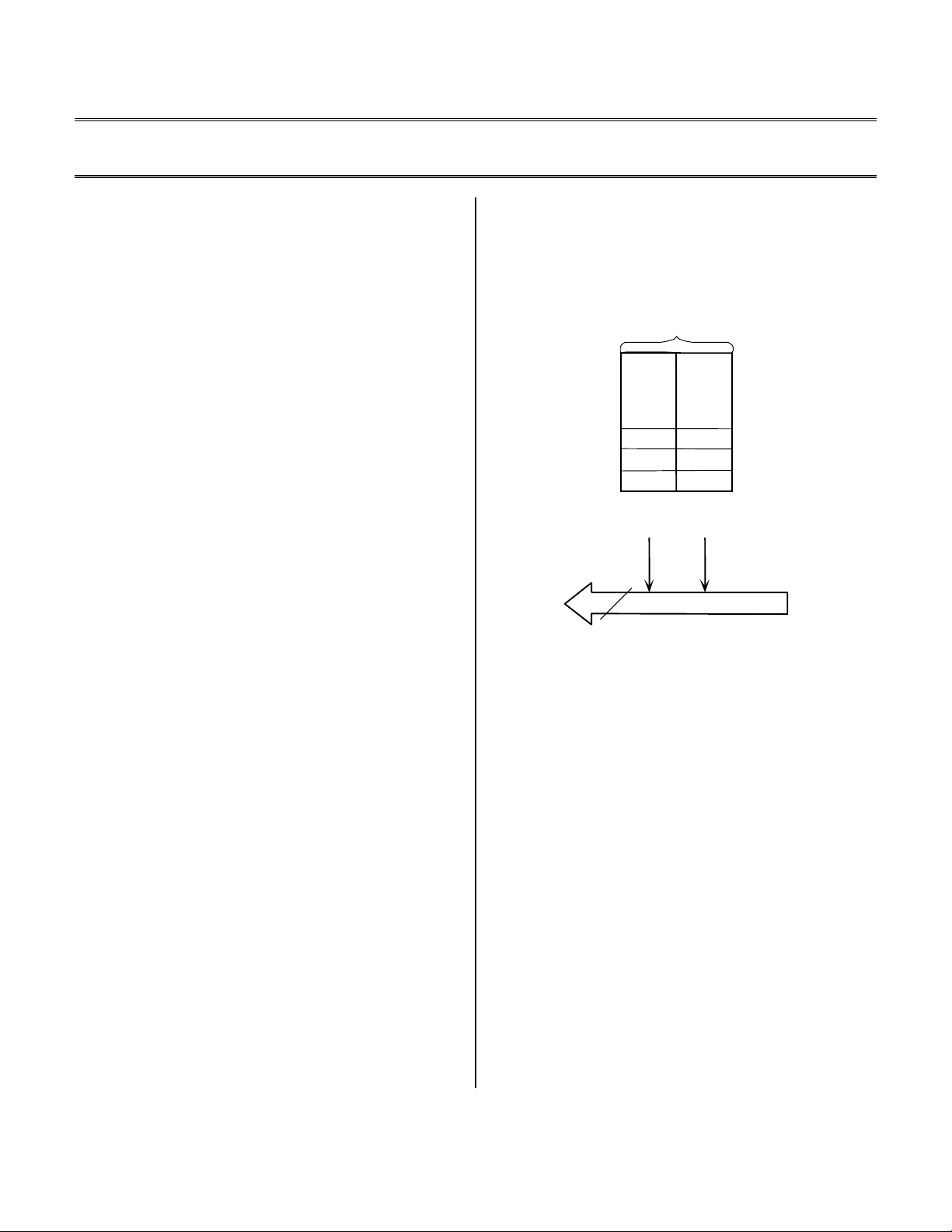
a
a
aa
Phone: (800) ANALOG-D, FAX: (781) 461-3010, EMAIL: dsp.support@analog.com, FTP: ftp.analog.com, WEB: www.analog.com/dsp
Engineer To Engineer Note EE-116
Technical Notes on using Analog Devices’ DSP components and development tools
SHARC SHORT WORD
DMA
Last Modified: 8/1/00
Introduction
The DMA Controller of the Analog Devices
SHARC® family processors does not
automatically dma 16 bit data from an external
memory to the SHARC’s internal memory,
however 16 bit data transfers can still easily be
accomplished. The following Engineer to
Engineer Note describes the method used to
move 16 bit data into the SHARC’s internal
memory and includes a sample code.
The external port and serial port DMA channels
have a packing capability allowing 16 bit data to
be packed to 32 bits. The 32 bit packed words
can be transferred to internal memory using
standard dma. Once the data is safely in internal
memory it can be accessed as short word
memory. Figure 1 illustrates how short words
are organized in normal word space. The least
significant short word address is generated by
arithmetically shifting the normal word address
to the left 1 bit. Arithmetically shifting the
normal word address to the left 1 bit and then
setting bit 0 to 1 generates the most significant
short word address. For example, if the normal
word address for a memory location were
0xC000 the short word address would be
0x18000 for the least significant word and
0x18001 for the most significant word.
ADSP-21065L SHARC User’s Manual. For
better understanding please see the chapters on
DMA and Memory in your User’s Manual.
32-bit Normal Wor ds
LSWMSW
.
.
.
ADDR 4
ADDR 2
ADDR 0
16-bi t
Short
Words
31-16
ADD R 2
ADDR 1
ADDR 0
.
.
.
ADDR 5
ADDR 3
ADDR 1
16-bit
Short
Word s
16
DATA
Figure 1. Short word addresses
All of this information can be found in the
ADSP-2106x SHARC User’s Manual or the
Copyright 2000, Analog Devices, Inc. All rights reserved. Analog Devices assumes no responsibility for customer product design or the use or application of customers’ products or
for any infringements of patents or rights of others which may result from Analog Devices assistance. All trademarks and logos are property of their respective holders. Information
furnished by Analog Devices Applications and Development Tools Engineers is believed to be accurate and reliable, however no responsibility is as sumed by Analog Devices
regarding the technical accuracy of the content provided in all Analog Devices’ Engineer-to-Engineer Notes.
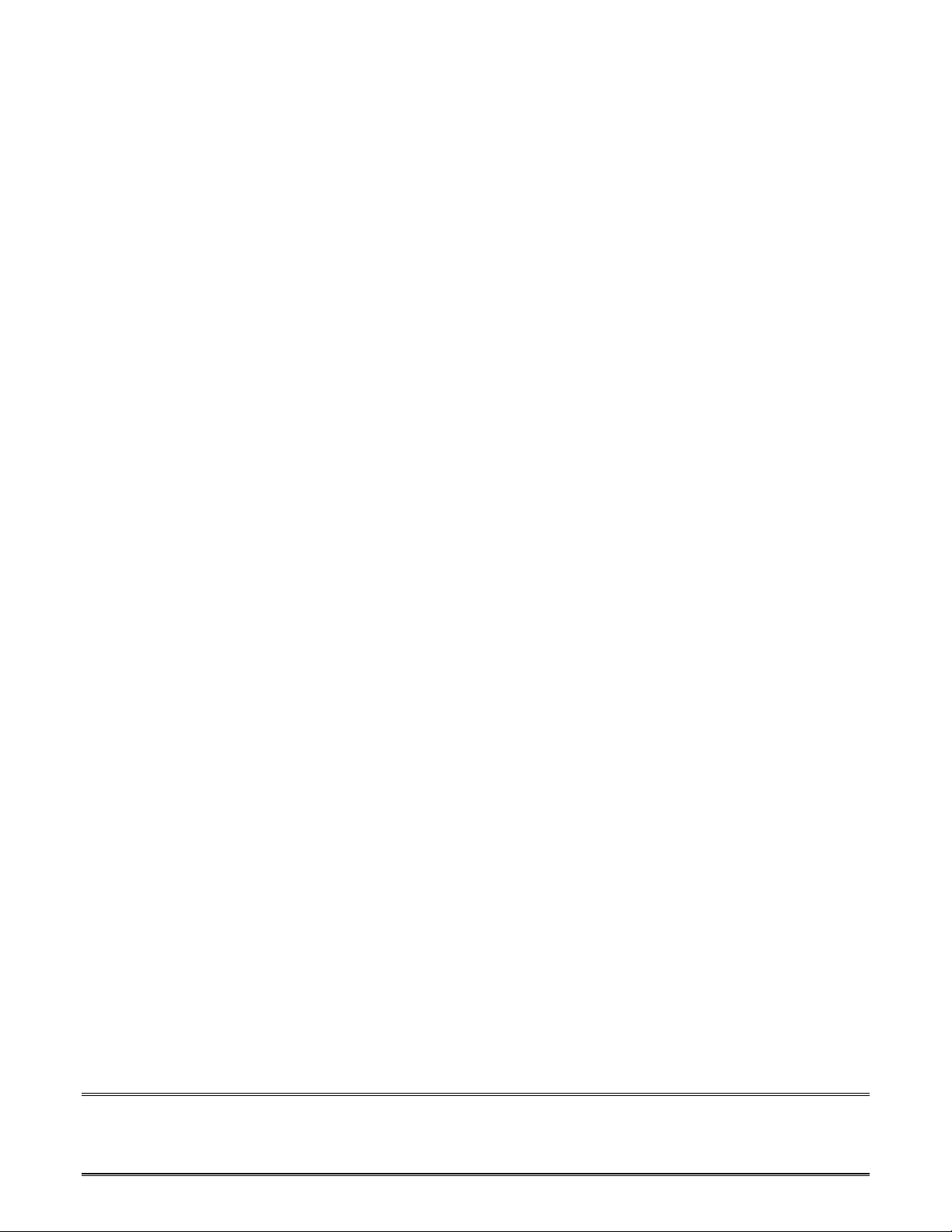
Listing1: dma.asm
/*
DMA.ASM ADSP-21065L EPORT 16/32 bit packing
GGL, Analog Devices, Inc. 7/19/00
This code is a simple example of getting 16 bit floating point data from SDRAM packing it to 32 bits
and using dma to get it into internal memory. Once in internal memory, the data is accessed as short
word and operated on and sent back to internal memory.
*/
#define N 4
#include "def21065l.h"
.section/DM seg_dmda;
.VAR dest[N];
/* This is your destination buffer in internal memory which resides in normal word space */
.section/DM seg_sdram;
.VAR source[2*N]=0x1111, 0x2222, 0x3333, 0x4444, 0xAAAA, 0xBBBB, 0xCCCC, 0xDDDD;
/* This is the sample 16 bit data from an external sdram */
.section/pm seg_rth;
/* This is the interrupt vector table */
Reserved_1: rti; nop; nop; nop;
Chip_Reset: idle; jump start; nop; nop;
Reserved_2: rti; nop; nop; nop;
stack_ov: rti; nop; nop; nop;
timerhi: rti; nop; nop; nop;
Vector: rti; nop; nop; nop;
IRQ2: rti; nop; nop; nop;
IRQ1: rti; nop; nop; nop;
IRQ0: rti; nop; nop; nop;
Reserved_3: rti; nop; nop; nop;
sport0r: rti; nop; nop; nop;
sport1r: rti; nop; nop; nop;
sport0t: rti; nop; nop; nop;
sport1t: rti; nop; nop; nop;
Reserved_4: rti; nop; nop; nop;
Reserved_5: rti; nop; nop; nop;
EP0: nop; nop; rti; nop;
EE-116 Page 2
Technical Notes on using Analog Devices’ DSP components and development tools
Phone: (800) ANALOG-D, FAX: (781)461-3010, EMAIL: dsp.support@analog.com, FTP: ftp.analog.com, WEB: www.analog.com/dsp
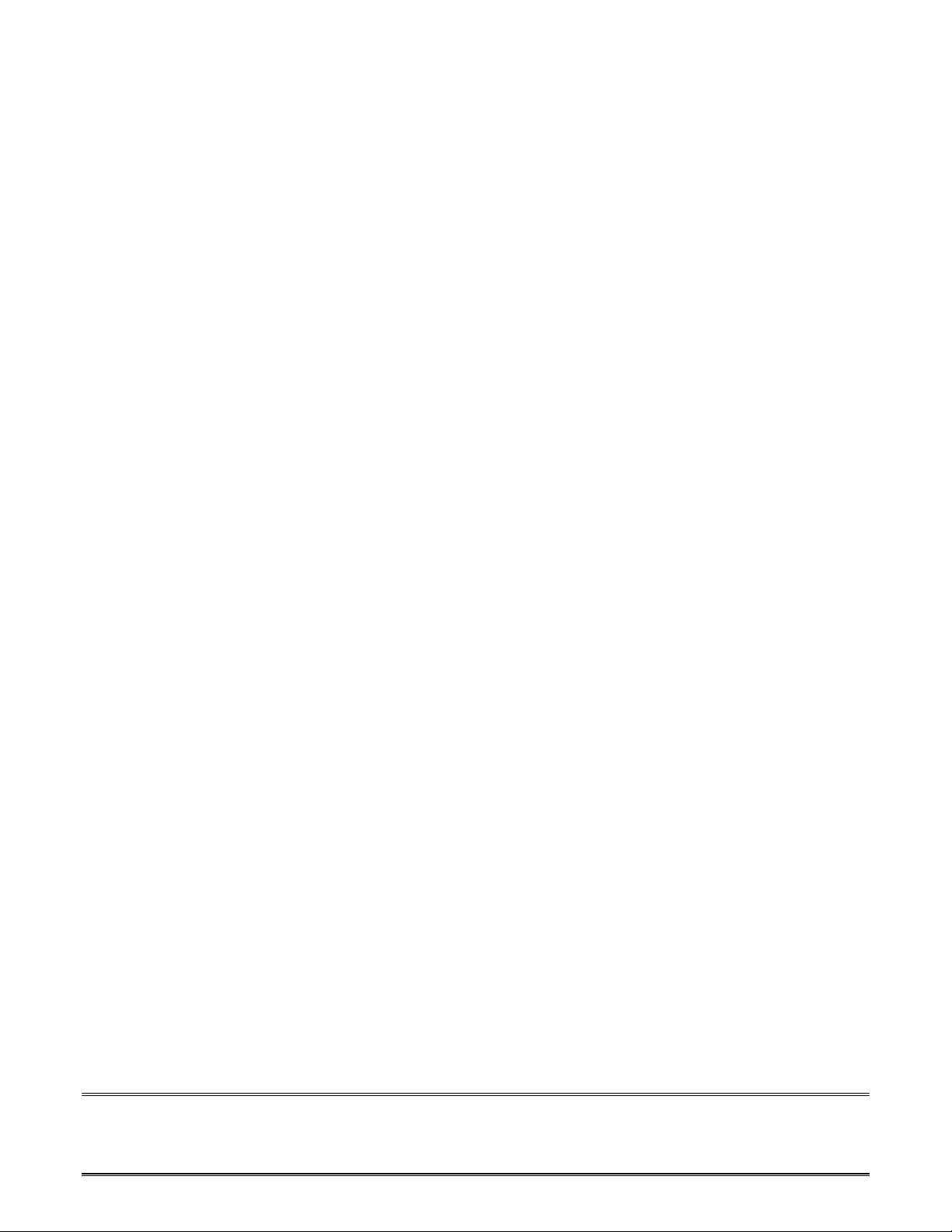
EP1: rti; nop; nop; nop;
.section/pm seg_pmco;
/*______________________start of main routine__________________________*/
start:
ustat1=937; /*refresh rate*/
dm(SDRDIV)=ustat1;
ustat1=dm(IOCTL); /*mask in SDRAM settings*/
bit set ustat1 SDPSS|SDBN2|SDBS3|SDTRP3|SDTRAS5|SDCL2|SDPGS256|SDDCK1;
dm(IOCTL)=ustat1; /*initialize sdram*/
/* enable interrupts here */
bit set MODE1 IRPTEN; /* enable global interrupts here */
bit set IMASK EP0I; /* enable eport interrupts here */
/* must set up II IM and C register in memory bufffer ; then enable the channel with DEN in DMAC */
/* dma channel 8 is external port buffer 0 */
r0=0; dm(DMAC0)=r0; /*clear DMA register*/
r0 = 1; dm(IMEP0) = r0; /* set DMA internal memory DMA modifier to 1*/
dm(EMEP0)=r0; /* set DMA external memory DMA
modifier to 1*/
r0 = N;dm(CEP0) = r0; /*set internal count to N */
r0=2*N; dm(ECEP0)=r0; /*set external DMA count to 2N */
r0=dest; dm(IIEP0) = r0; /* Write internal index pointer for dest */
r0=source; dm(EIEP0)=r0; /*Write External DMA buffer Index pointer for source */
r0=0x0241; dm(DMAC0)=r0; /* enable DMA channel, master mode, 16/32bit
packing */
wait1: idle;
/* when this dma completes you will have the 16 bit words that were stored in SDRAM
packed to 32 bit words in external memory */
/* the ISR only has an RTI in it, so after the dma is complete
the program flow will continue in the next instruction */
/* find the first address of the data buffer and convert it to short word */
r0=dest;
EE-116 Page 3
Technical Notes on using Analog Devices’ DSP components and development tools
Phone: (800) ANALOG-D, FAX: (781)461-3010, EMAIL: dsp.support@analog.com, FTP: ftp.analog.com, WEB: www.analog.com/dsp