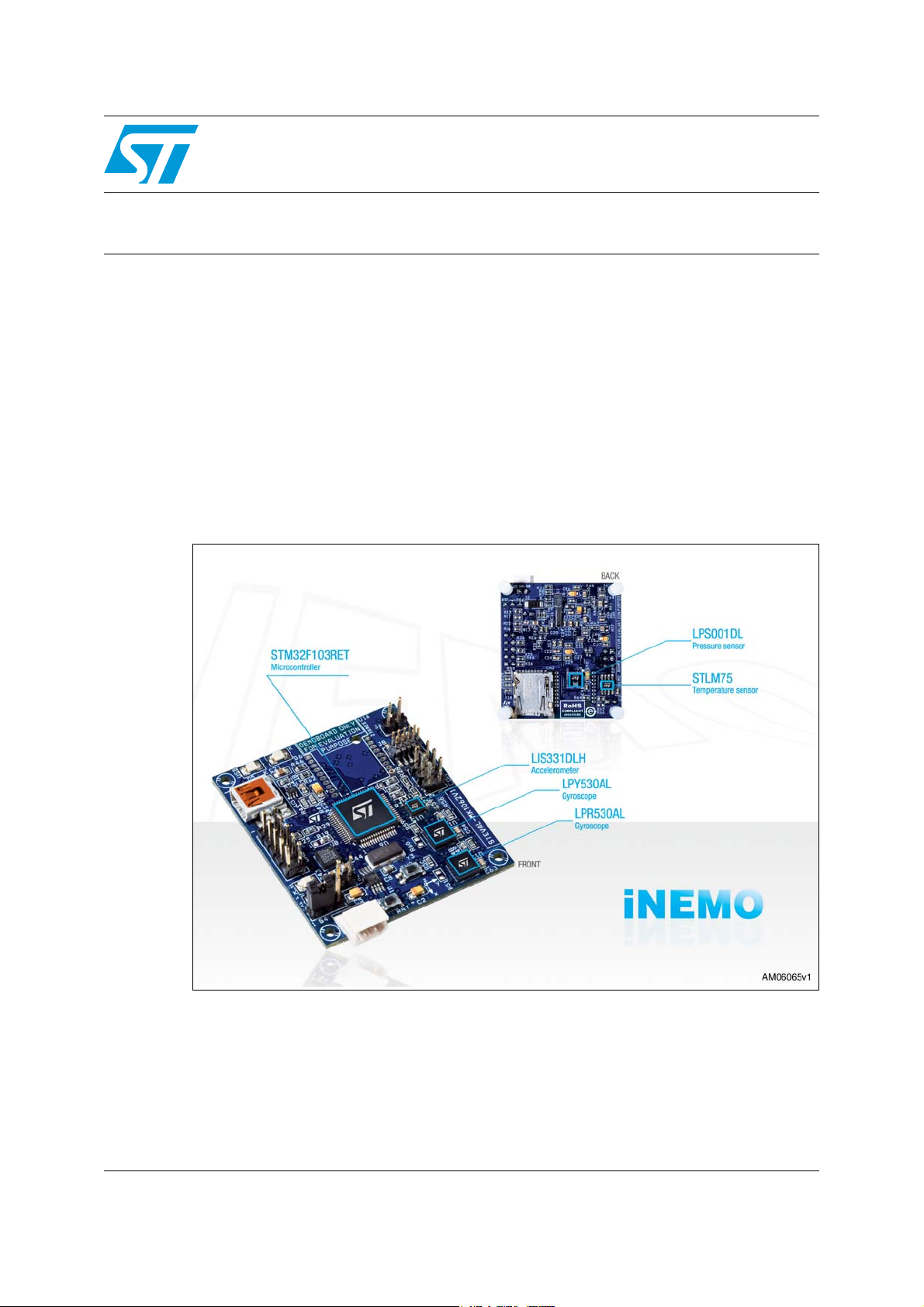
Wrapper in Visual Studio for the STEVAL-MKI062V1
Introduction
AN3144
Application note
This application note describes how to interface a Microsoft® Visual Studio® project with the
dynamic link library (DLL) of the application programming interface (API) of the iNEMO
software development kit (SDK).
The DLL object handles the hardware communication interfacing and is an easy way to
achieve (soft) real-time performance. The use of DLLs helps with the modularization of
code, code reuse, efficient memory use, and reduction of disk space. Typically, this is
preferred when you want to access the iNEMO
MKI062V1) capabilities directly from a software project without accessing the
microcontroller firmware.
Figure 1. iNemo
TM
platform
TM
demonstration board (STEVAL-
TM
July 2010 Doc ID 17013 Rev 1 1/15
www.st.com

Contents AN3144
Contents
1 Getting started . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 3
1.1 Power-up . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 3
1.2 Notes on Microsoft Visual Studio version . . . . . . . . . . . . . . . . . . . . . . . . . . 3
1.3 iNEMO connection . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 4
1.4 Releasing the application . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 5
2 Application code . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
2.1 Package description . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
2.2 Dynamic link library loading . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
2.2.1 Loading the iNEMO_SDK.DLL . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
2.2.2 How to use the DLL functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 7
2.3 iNEMO functions in detail . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 8
2.3.1 Connecting the PC to iNEMO . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 8
2.3.2 Disconnecting the connected iNEMO device . . . . . . . . . . . . . . . . . . . . . . 9
2.3.3 Checks the iNEMO device connection state . . . . . . . . . . . . . . . . . . . . . . 9
2.3.4 Gets the firmware version . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 9
2.3.5 Starting data acquisition . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10
2.3.6 Stopping the current acquisition . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10
2.3.7 Getting a data sample . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11
2.3.8 Getting the internal buffer usage of the FIFO . . . . . . . . . . . . . . . . . . . . 12
3 References and related materials . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13
4 Revision history . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14
2/15 Doc ID 17013 Rev 1

AN3144 Getting started
1 Getting started
1.1 Power-up
The STEVAL-MKI062V1 board can be supplied by USB. Before connecting the board to a
USB port, configure switch S4 as described in the user manual for the board (UM0752).
The STEVAL-MKI062V1 PC software must be pre-installed on the computer before using
the C++ code described in this application note, and it is necessary to download iNEMO
application 1.0.8 or higher.
1.2 Notes on Microsoft Visual Studio version
The software code described in this application note is compatible with the following
versions of the development environment:
● Microsoft Visual Studio 2005
● Microsoft Visual Studio .NET 2003
● Microsoft Visual Studio .NET 2002
As a guideline, users can follow these steps to make a project capable of managing the
iNEMO DLL from native C++ code:
1. Start Visual Studio.
2. In the File menu, click New, and then Project.
3. If you are using Visual C++
Project Types, and then click Win32 Console Project or Win32 Project under
Templates. If you are using Visual C++ 2005, click Visual C++ under Project Types, and
then click Win32 Project under Templates.
4. In the Name text box, type iNEMO_Cpp_Wrapper, and then click OK.
5. If you are using Visual C++ .NET 2003 or Visual C++ 2005, click Finish. If you are using
Visual C++ .NET 2002, click Application Settings, click Console Application, and then
click Finish.
6. Open the iNEMO_Cpp_Wrapper file in Code view.
®
.NET 2002 or 2003, click Visual C++ Projects under
If you receive the error message “Run-Time Check Failure #0”, the value of ESP was not
properly saved across a function call. This is usually a result of calling a function declared
with one calling convention with a function pointer declared using a different calling
convention. When you run the debug build of your application, you can resolve this by the
following steps:
1. In Solution Explorer, right-click on your ProjectName, and then click Properties. The
ProjectName Property Pages dialog box appears.
2. In the left pane of the ProjectName Property Pages dialog box, click Configuration
Properties.
3. Under Configuration Properties, click C/C++, and then click Code Generation.
4. Set the Basic Runtime Checks property to Default.
5. In the ProjectName Property Pages dialog box, click OK.
6. In the File menu, click Save All to save all of the files.
Doc ID 17013 Rev 1 3/15

Getting started AN3144
1.3 iNEMO connection
Once the iNEMO demonstration board is connected to a USB port, it is identified by the PC
through the virtual COM port driver, and the PC assigns a port number to the iNEMO board.
To identify which port number has been assigned by the PC, follow these steps:
1. On the PC desktop, right click on the My Computer icon and click on Manage (see
Figure 2).
2. In Computer Management under System Tools, click on Device Manager to see all the
devices connected to the PC in the right-hand pane.
3. In the right-hand pane, click the “+” next to Ports (COM & LPT) to see the list of
available ports. In this list you should find the STEVAL-MKI062V1 iNEMO Platform
(COMx) string (see Figure 3).
The string containing the COM port number assigned to the board is used by the source
code described in Section 2.3.1: Connecting the PC to iNEMO.
Note: The COM port number assigned is valid for one session only; when the board is unplugged
and plugged in again (especially into a new USB port) the COM port number assigned could
be different. It should therefore be checked again using the procedure described above.
Figure 2. Identifying the COM port used by the iNEMO board - first step
4/15 Doc ID 17013 Rev 1
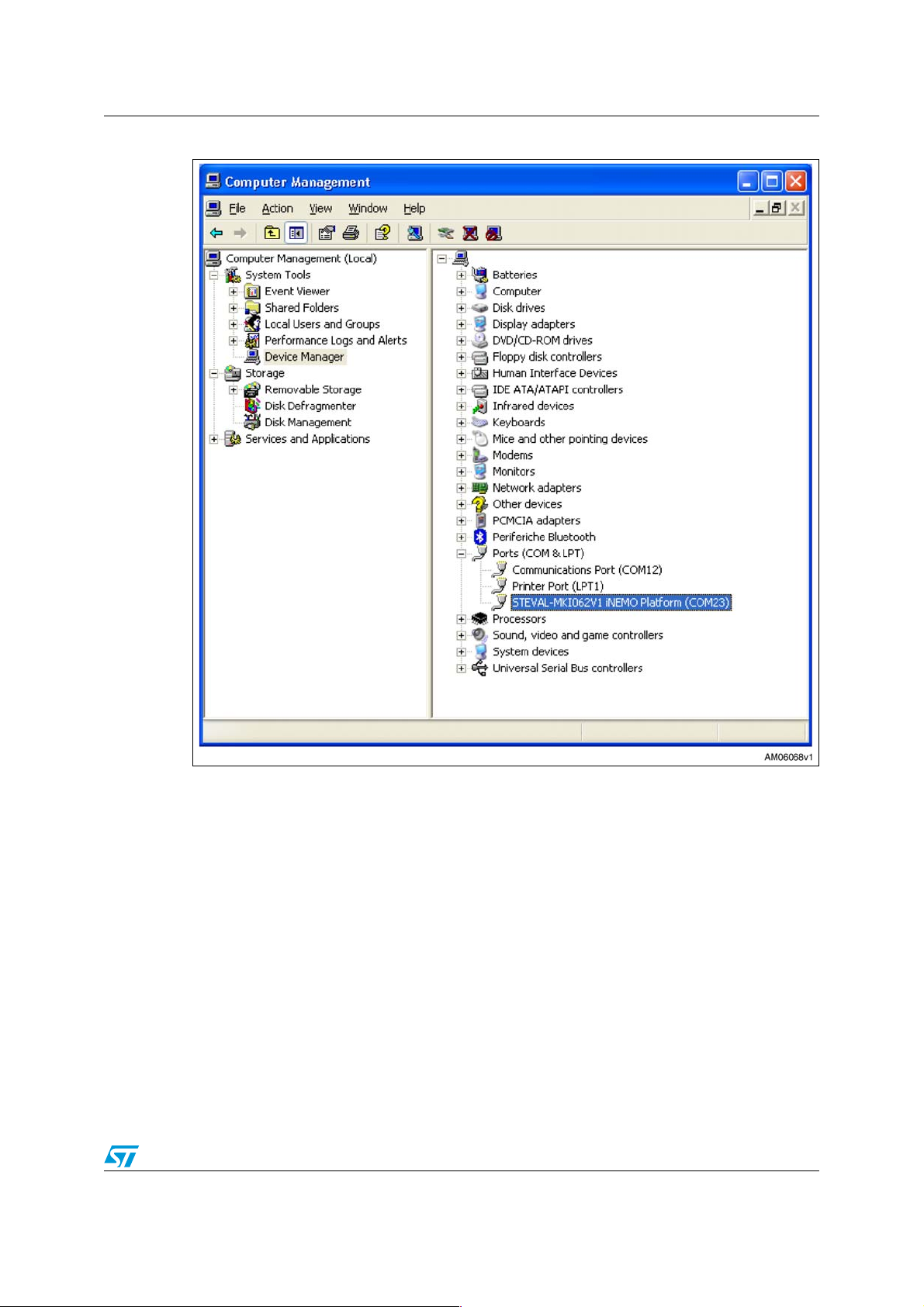
AN3144 Getting started
Figure 3. Identifying the COM port used by iNEMO board - second step
1.4 Releasing the application
You can freely release your own application which is linked with iNEMO DLL. Because the
iNEMO_SDK.dll is dependent on the PL_001.dll, both DLLs must be located in the same
folder. It is recommended to have the two DLLs in the application folder to avoid conflicts
with different versions.
Doc ID 17013 Rev 1 5/15