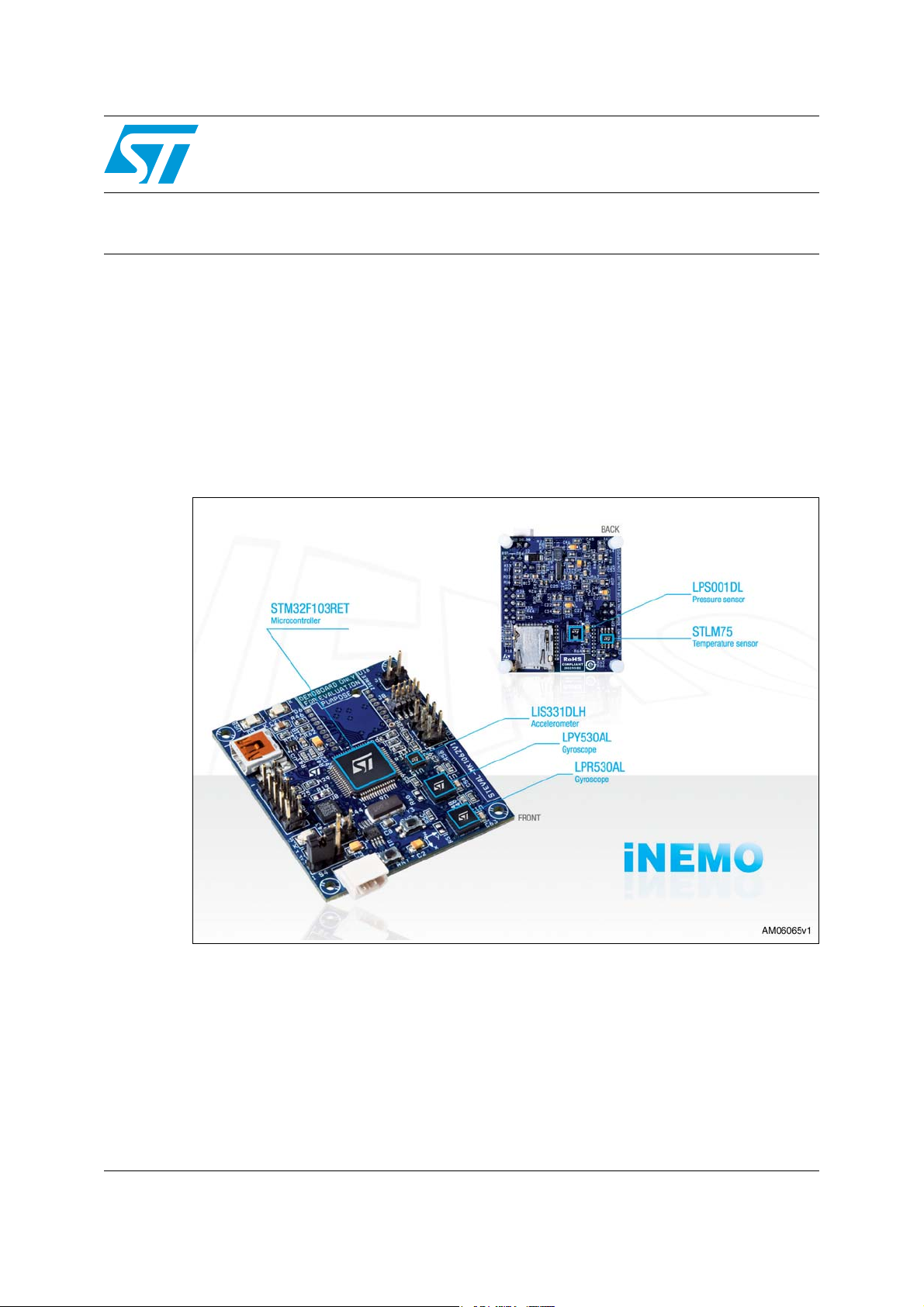
AN3138
Application note
Wrapper in MATLAB for the STEVAL-MKI062V1
Introduction
This application note provides information on the use of the application programming
interface (API) of the iNEMO™ software development kit (SDK), which provides easy-to-use
function calls to obtain data from the sensors or to change settings. The dynamic link library
(DLL) object handles the hardware communication interfacing and is an easy way to achieve
(soft) real-time performance. Typically, this is preferred when you want to access the iNEMO
board (STEVAL-MKI062V1) capabilities directly in the MathWorks™ MATLAB
software.
®
application
Figure 1. iNemo
TM
platform
January 2010 Doc ID 16985 Rev 1 1/16
www.st.com
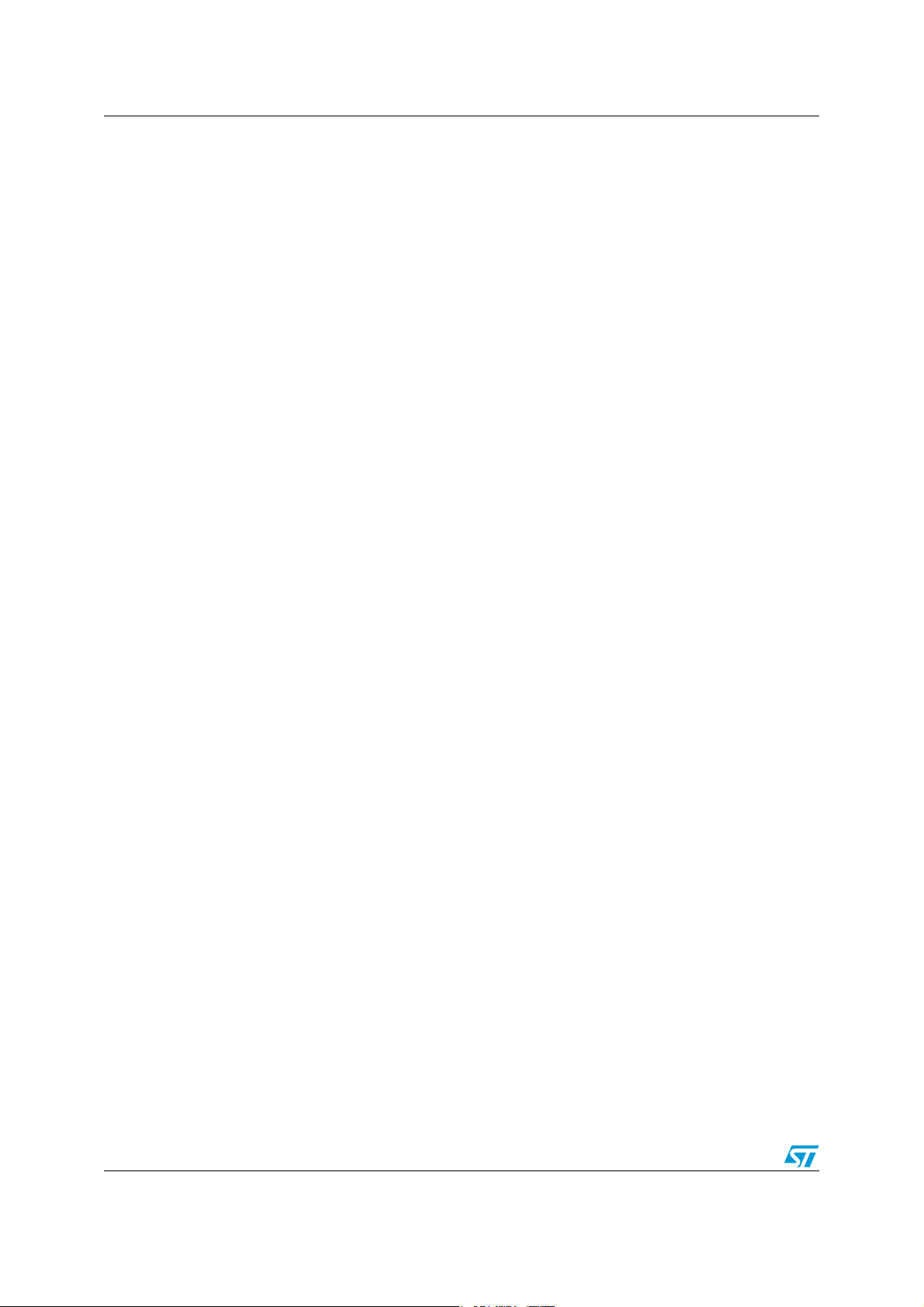
Contents AN3138
Contents
1 Getting started . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 3
1.1 Power-up . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 3
1.2 MATLAB version . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 3
2 Application code . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 4
2.1 Package description . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 4
2.2 Generic MATLAB script usage . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 4
2.3 Main functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 5
2.3.1 Connect . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 5
2.3.2 Start data acquisition . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 7
2.3.3 Acquire data . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 9
2.3.4 Stop acquisition . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 9
3 References and related materials . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10
Appendix A MATLAB script code . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11
4 Revision history . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 15
2/16 Doc ID 16985 Rev 1
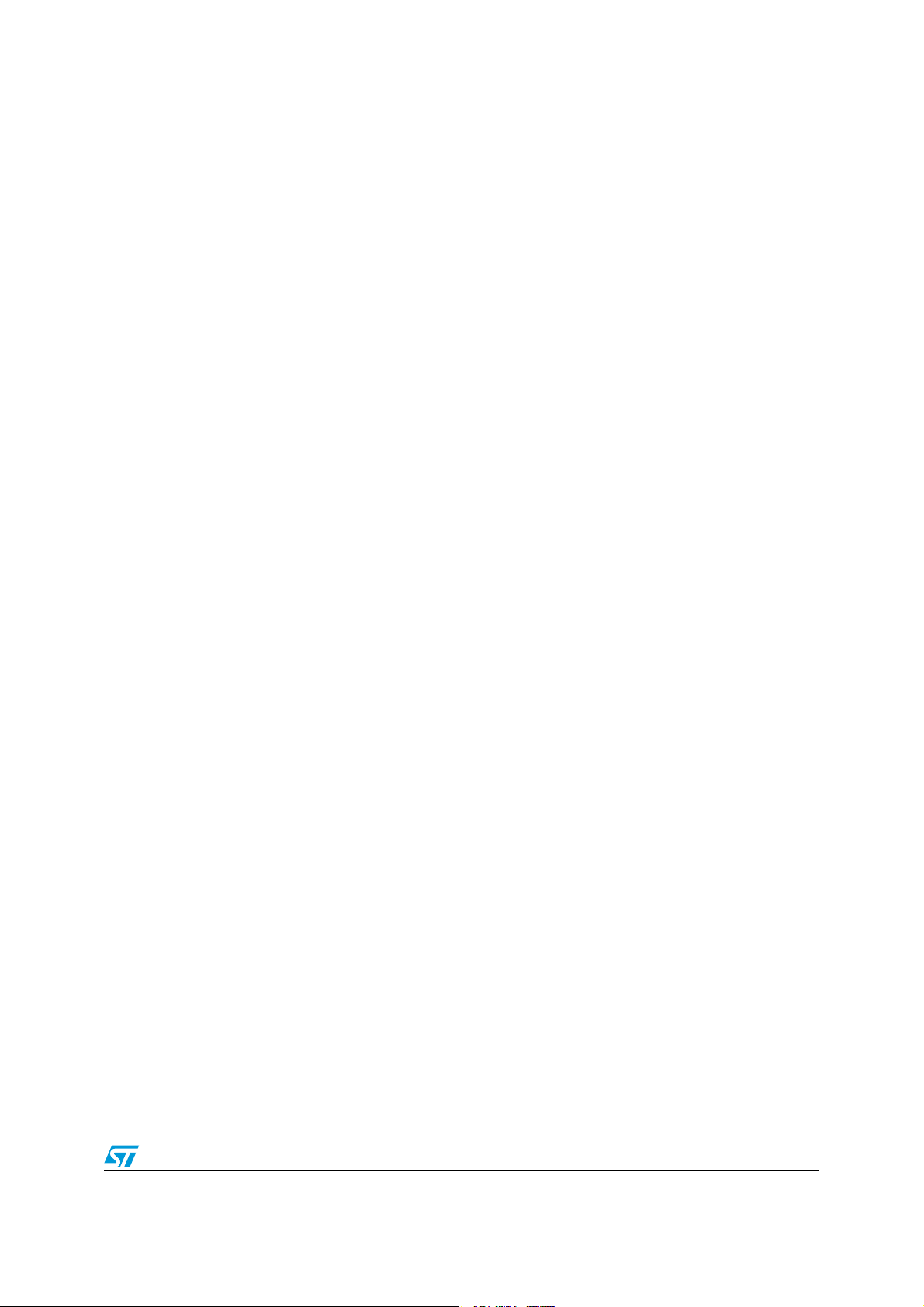
AN3138 Getting started
1 Getting started
1.1 Power-up
The STEVAL-MKI062V1 board can be supplied by USB. Before connecting the board to a
USB port, configure switch S4 as described in the user manual of the board (UM0752).
The STEVAL-MKI062V1 software must be pre-installed on the computer before to using the
MATLAB script described in this application note.
1.2 MATLAB version
MathWorks MATLAB 7.8 (release R2009a and higher) is compatible with the iNEMO SDK
object. Older versions have not been tested.
Doc ID 16985 Rev 1 3/16
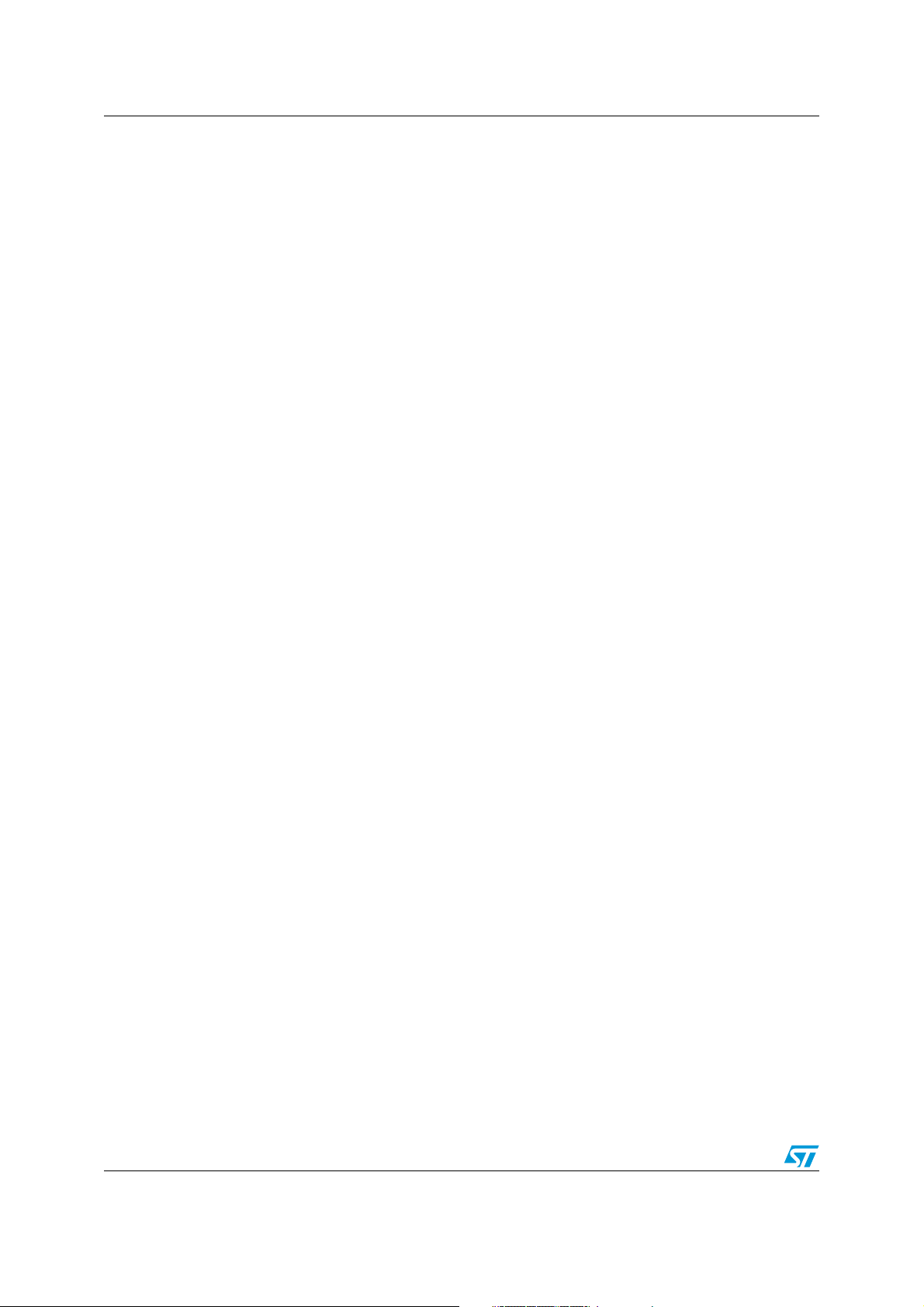
Application code AN3138
2 Application code
2.1 Package description
Example codes are provided in the subfolder:
\STMicroelectronics\iNEMO Application\Redist
(the folder name could be different on your computer)
This subfolder contains:
● iNEMO_SDK.chm
– help file for the software development kit
● iNEMO_SDK.dll
– dynamic link library for the SDK
● iNEMO_SDL.h
– header file for the iNEMO SDK dll
● iNEMO_SDK_Wrapper.cs
– wrapper file in C#
● PL_001.dll - PL_001
– dynamic link library
● iNEMO_easy_script.m
– MATLAB example (included in Appendix A)
The iNEMO_easy_script.m file contains all the step-by-step instructions needed to use the
iNEMO SDK API in MATLAB. The explanations in the sections that follow may be used as a
guide for this file.
2.2 Generic MATLAB script usage
To load the dynamic link library within MATLAB, the load library instruction must be used
with the syntax:
[notfound, warnings] = loadlibrary('shrlib', 'hfile')
where the hfile and shrlib are the filenames of the DLL and header files, respectively (names
are case-sensitive). The name used in the load library must be in the same case as the file
on your system. In the present case:
[notfound, warnings] = loadlibrary('iNEMO_SDK.dll', 'iNEMO_SDK.h');
Once the iNEMO_SDK object is loaded, its functions can be listed easily with the
instructions:
libfunctions('iNEMO_SDK')
or
libfunctionsview('iNEMO_SDK')
Using one of these functions, the MATLAB displays the window in Figure 2 with all the
available functions that can be executed by using the calllib function:
[ret_1, …] = calllib('iNEMO_SDK', function_name, arg_1, arg_2, …);
4/16 Doc ID 16985 Rev 1
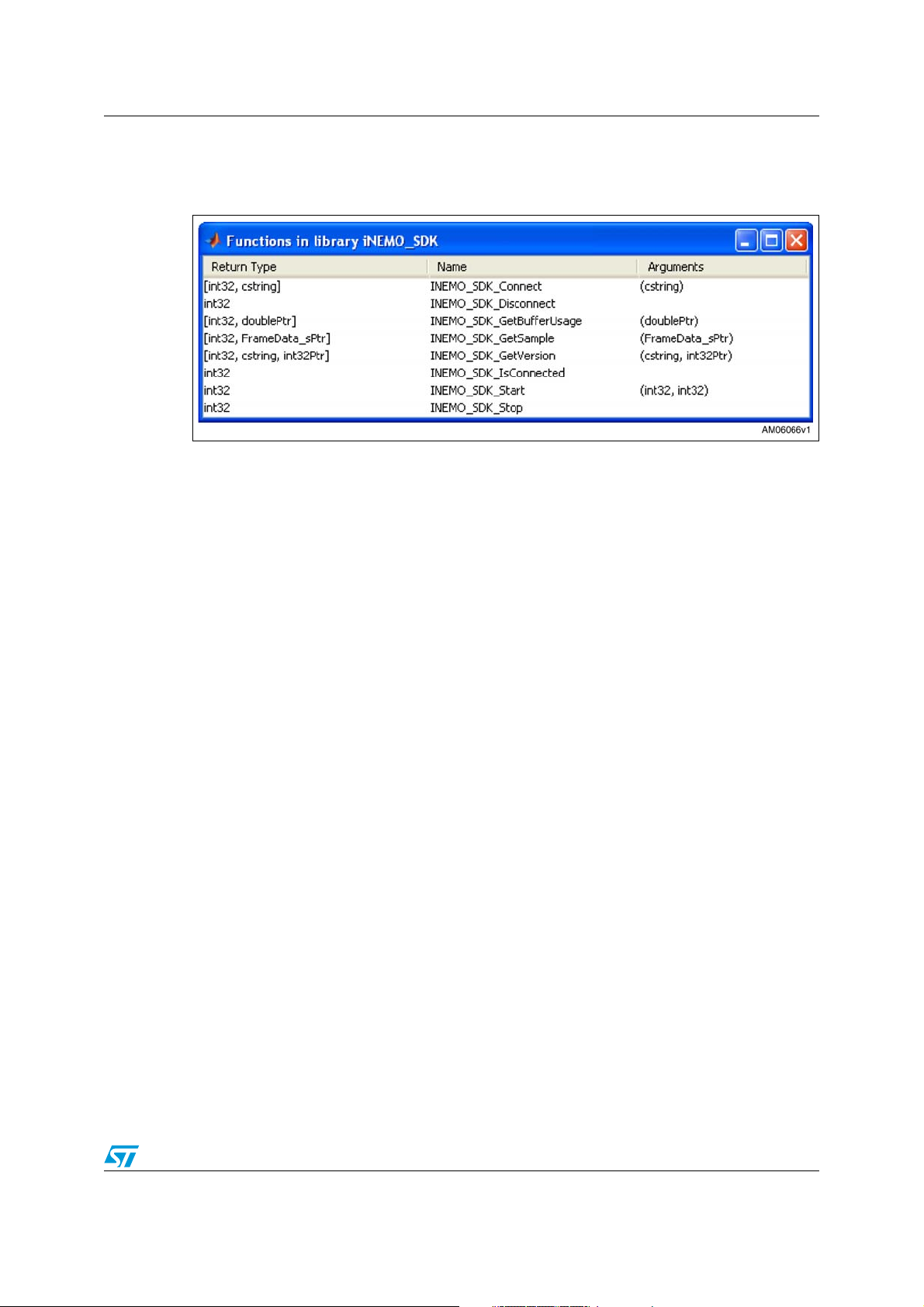
AN3138 Application code
The function_name (case-sensitive) should be one of the functions listed in Figure 2. arg_n
are the arguments of the functions, and ret_n are the function results.
Figure 2. Window displaying accessible functions
Finally, to release the iNEMO_SDK from the MATLAB environment, the following function
call must be used:
Unloadlibrary('iNEMO_SDK')
Note: Improper use of function sequence calls may cause MATLAB to release the object
incorrectly, resulting in error messages or unexpected behavior of the MATLAB
environment. Shutting down MATLAB will always release the iNEMO_SDK object.
Moreover, to restart the iNEMO board in the initial state, it is recommended to disconnect
and then reconnect the board.
2.3 Main functions
The iNEMO software development kit (SDK) allows implementation of the following actions:
● Connect the iNEMO board (STEVAL-MKI062V1) through USB virtual COM
● Start data acquisition
● Acquire data
● Stop acquisition
● Disconnect the iNEMO board
2.3.1 Connect
Once the iNEMO_SDK library is loaded, the first instruction to be used is:
calllib('iNEMO_SDK', 'INEMO_SDK_Connect', sCOM);
where sCOM should be a string in the format 'PL_001:COMx, BD115200'. COMx indicates
the virtual COM port used by the iNEMO_SDK.
Doc ID 16985 Rev 1 5/16