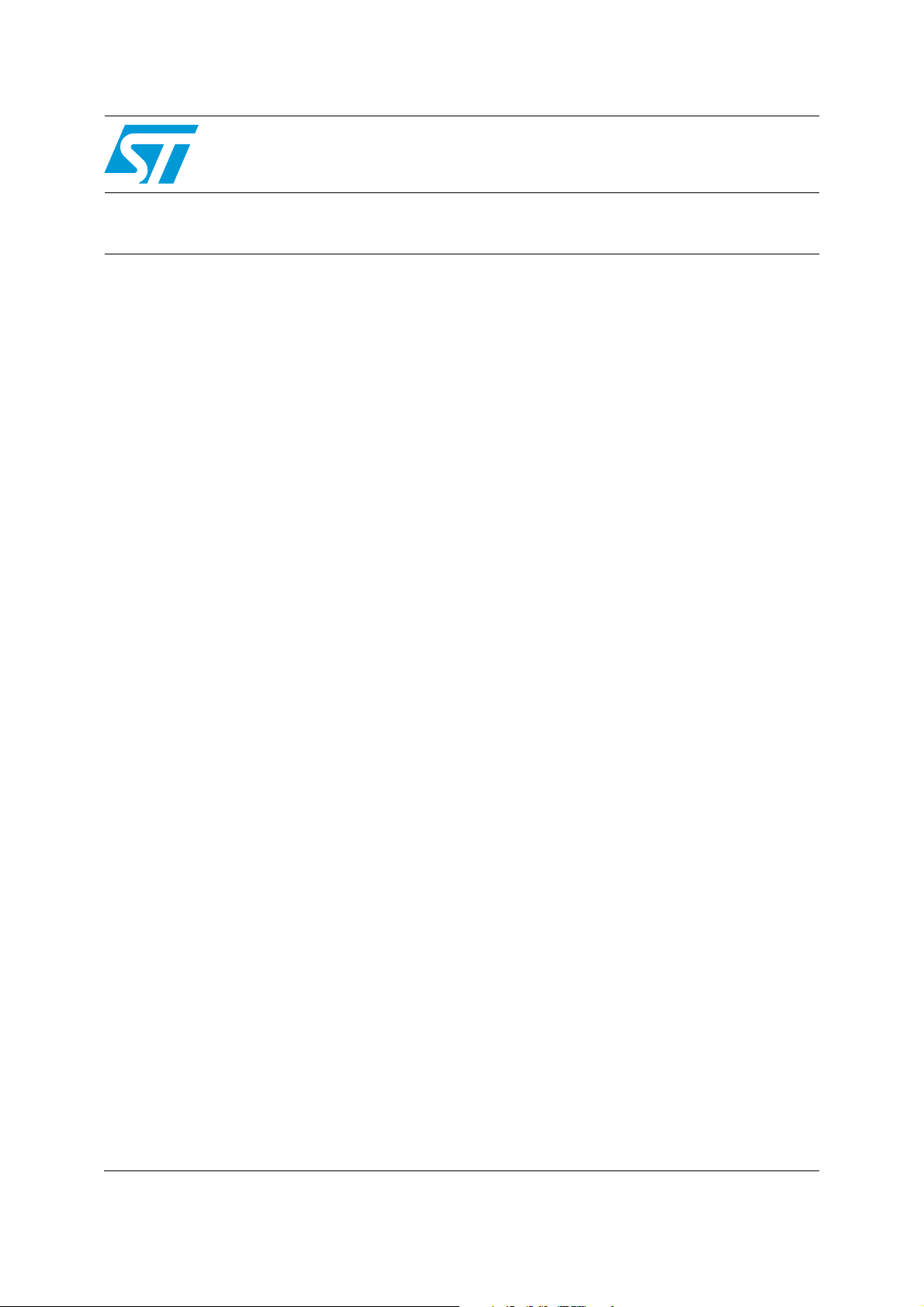
AN2931
Application note
Implementing the ADPCM algorithm
in high-density STM32F103xx microcontrollers
Introduction
This application note describes the ADPCM audio firmware codec, and provides a
demonstration firmware showing how to play ADPCM files using the high-density
STM32F103xx I
2
S feature with an external DAC.
This application note is based on “AN2739: How to use the high-density STM32F103xx
microcontroller to play audio files with an external I
the SPI, I
2
S and the external DAC will be skipped here because they are already explained
2
S audio codec”. The features linked to
in AN2739. It is therefore highly recommended to refer to AN2739 to fully understand this
application note.
April 2009 Doc ID 15349 Rev 2 1/15
www.st.com

Contents AN2931
Contents
1 ADPCM algorithm . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 5
1.1 General overview . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 5
1.2 ADPCM algorithm implementation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 5
1.3 ADPCM algorithm functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
1.3.1 ADPCM_Encode function . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
1.3.2 ADPCM_Decode function . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
2 Implementation example . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 8
2.1 Description . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 8
2.2 ADPCM file generation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 8
2.3 ADPCM file loading . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 9
2.4 ADPCM file playing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11
3 Conclusion . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13
4 Revision history . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14
2/15 Doc ID 15349 Rev 2

AN2931 List of tables
List of tables
Table 1. ADPCM algorithm functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
Table 2. ADPCM_Encode function . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
Table 3. ADPCM_Decode function . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 7
Table 4. Document revision history . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14
Doc ID 15349 Rev 2 3/15

List of figures AN2931
List of figures
Figure 1. ADPCM file generator . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 8
Figure 2. DFU File Manager . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10
Figure 3. DFU File Manager source browser . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10
Figure 4. DfuSe software . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11
4/15 Doc ID 15349 Rev 2
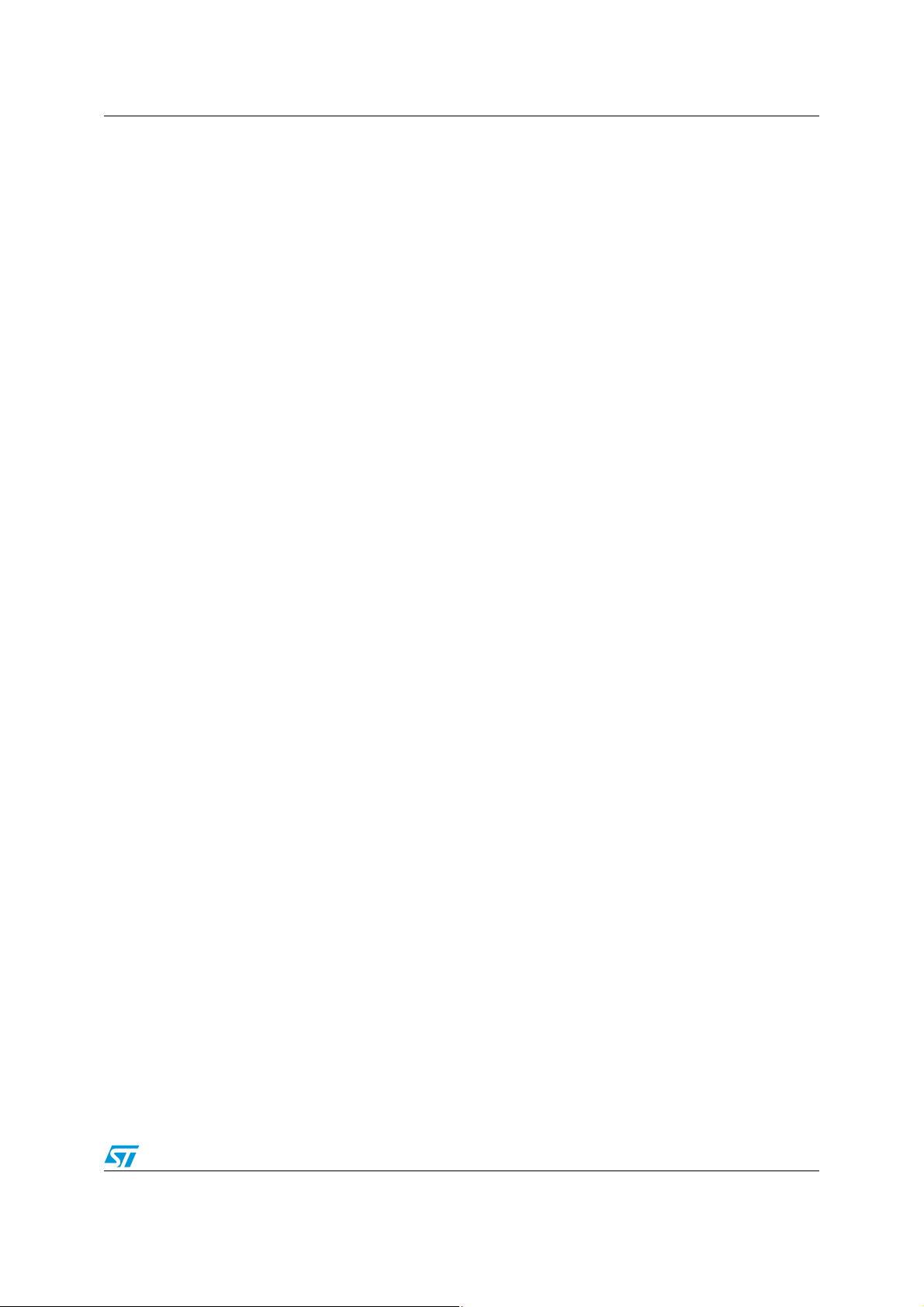
AN2931 ADPCM algorithm
1 ADPCM algorithm
1.1 General overview
Adaptive differential pulse-code modulation, or simply ADPCM, is an audio algorithm for
waveform coding, which consists in predicting the current signal value from previous values,
and transmitting only the difference between the real and the predicted value.
In plain pulse-code modulation (PCM), the real or actual signal value is transmitted.
The advantage of ADPCM is that the difference between the predicted signal value and the
actual signal value is usually quite small, which means it can be represented using fewer
bits than the corresponding PCM value.
Depending on the desired quality and compression ratio, the differential signal is quantized
using 4 (2 bit), 8 (3 bit), 16 (4 bit) or 32 (5 bit) levels.
Many implementations of the ADPCM algorithm exist. They differ by the quantization and
the prediction patterns.
In this application note, we provide a 4-bit quantization algorithm, developed by the
Interactive Multimedia Association (IMA), IMA ADPCM.
IMA ADPCM was chosen for several reasons:
● it can operate at different sampling rates between 8 kHz and 44.10 kHz
● it guarantees good quality with low CPU usage and memory size requirements
● it has widespread implementations, for instance in the Windows and MAC operating
systems
The IMA ADPCM algorithm is fully described in a document published by the IMA Digital
Audio Focus and Technical Working Groups: “Recommended Practices for Enhancing
Digital Audio Compatibility in Multimedia Systems” revision 3.
1.2 ADPCM algorithm implementation
The IMA ADPCM algorithm provided in this application note is used to encode audio files
that have the following specification:
● Audio format: PCM
● Audio sample size: 16 bits
● Channels: 1 (mono)
● Audio sample rate: from 8 kHz to 44.1 kHz
Each 16-bit PCM sample is encoded into a 4-bit ADPCM sample, which gives a
compression rate equal to ¼.
The implementation of the IMA ADPCM algorithm consists in two functions, one that codes
and the other that decodes the audio samples.
The ADPCM firmware is composed of two files:
a) adpcm.c: it contains the source code of the two ADPCM functions that perform the
coding and decoding.
b) adpcm.h: it is the header file of adpmc.c. It should be included in the files where
the ADPCM functions are called.
Doc ID 15349 Rev 2 5/15