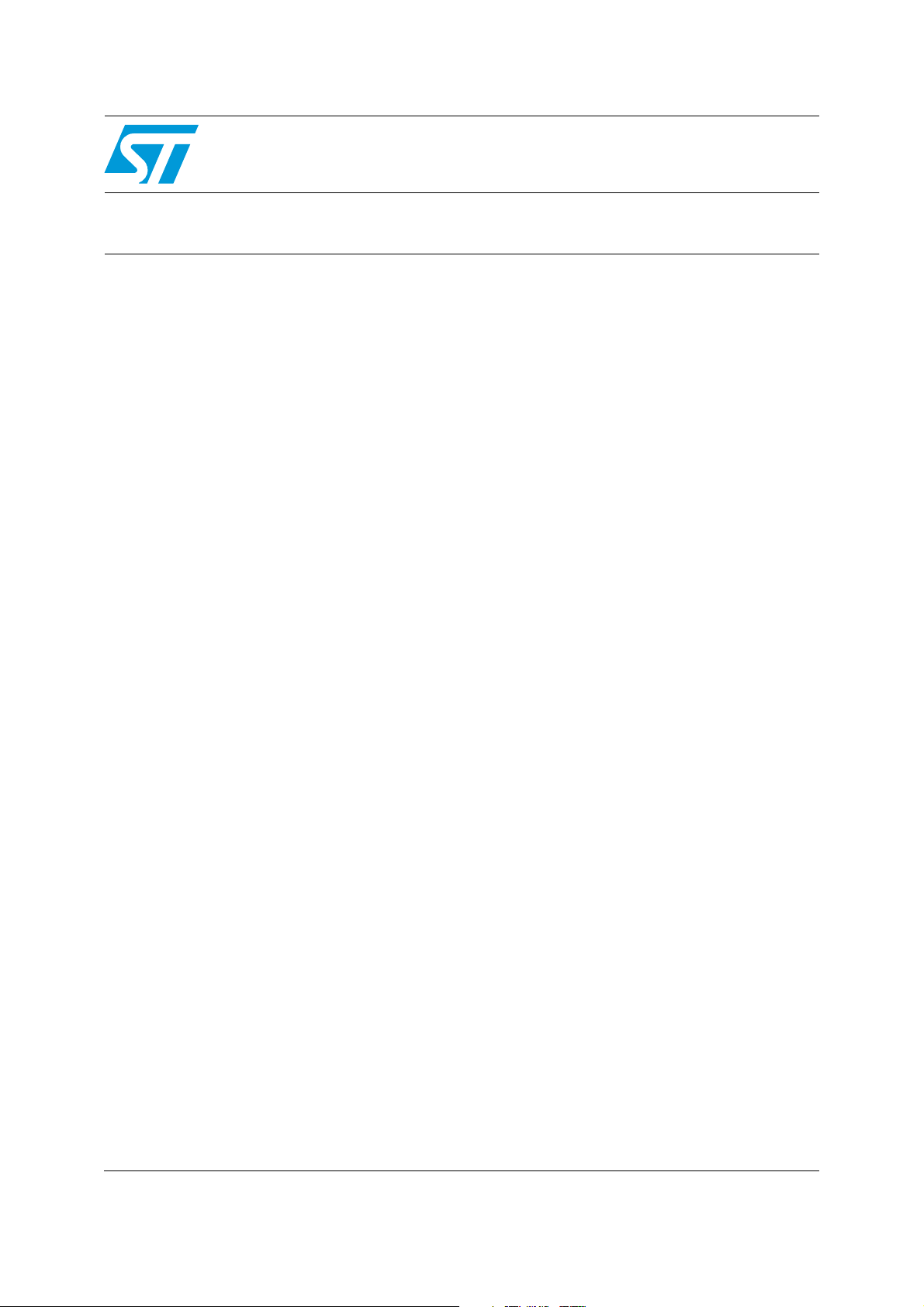
AN2557
Application note
STM32F10x in-application programming
using the USART
Introduction
An important requirement for most Flash-memory-based systems is the ability to update
firmware when installed in the end product. This ability is referred to as in-application
programming (IAP). The purpose of this application note is to provide general guidelines for
creating an IAP application. The STM32100B-EVAL, STM32100E-EVAL, STM3210B-EVAL,
STM3210E-EVAL and STM3210C-EVAL boards were used to validate the IAP driver.
The STM32F10x microcontroller can run user-specific firmware to perform IAP of the
microcontroller-embedded Flash memory. This feature allows the use of any type of
communication protocol for the reprogramming process (such as CAN, USART, USB).
USART is the example used in this application note.
October 2010 Doc ID 13588 Rev 8 1/16
www.st.com
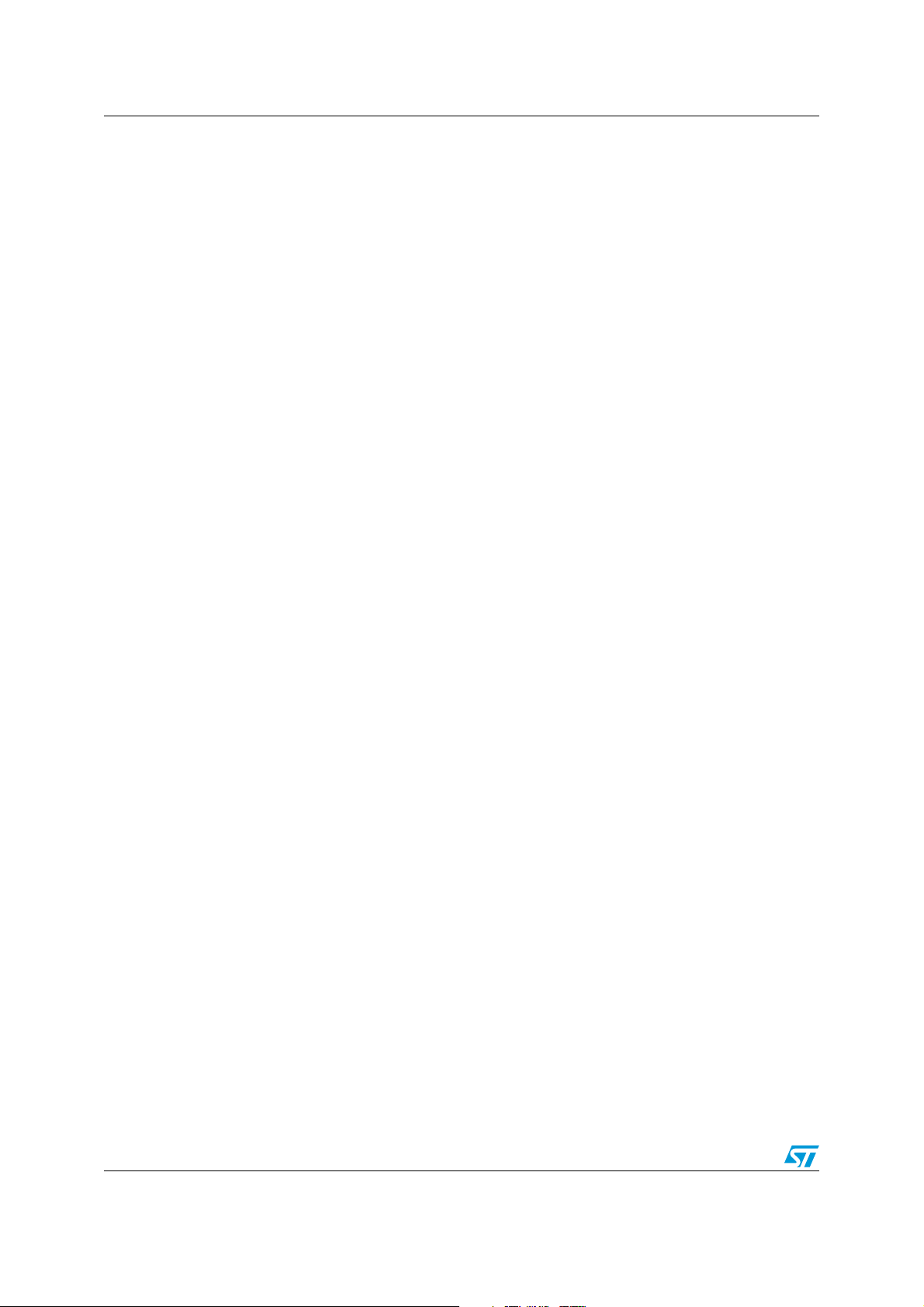
Contents AN2557
Contents
1 IAP overview . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 5
1.1 Principle . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 5
1.2 IAP driver description . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 5
2 Running the IAP driver . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 8
2.1 HyperTerminal configuration . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 8
2.2 Executing the IAP driver . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 9
3 IAP driver menu . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10
3.1 Download image to the internal Flash memory . . . . . . . . . . . . . . . . . . . . 10
3.2 Upload image from the internal Flash memory . . . . . . . . . . . . . . . . . . . . 11
3.3 Execute the new program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11
3.4 Disabling the write protection . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11
4 STM32F10x IAP implementation summary . . . . . . . . . . . . . . . . . . . . . . 12
5 User program conditions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14
6 Revision history . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 15
2/16 Doc ID 13588 Rev 8
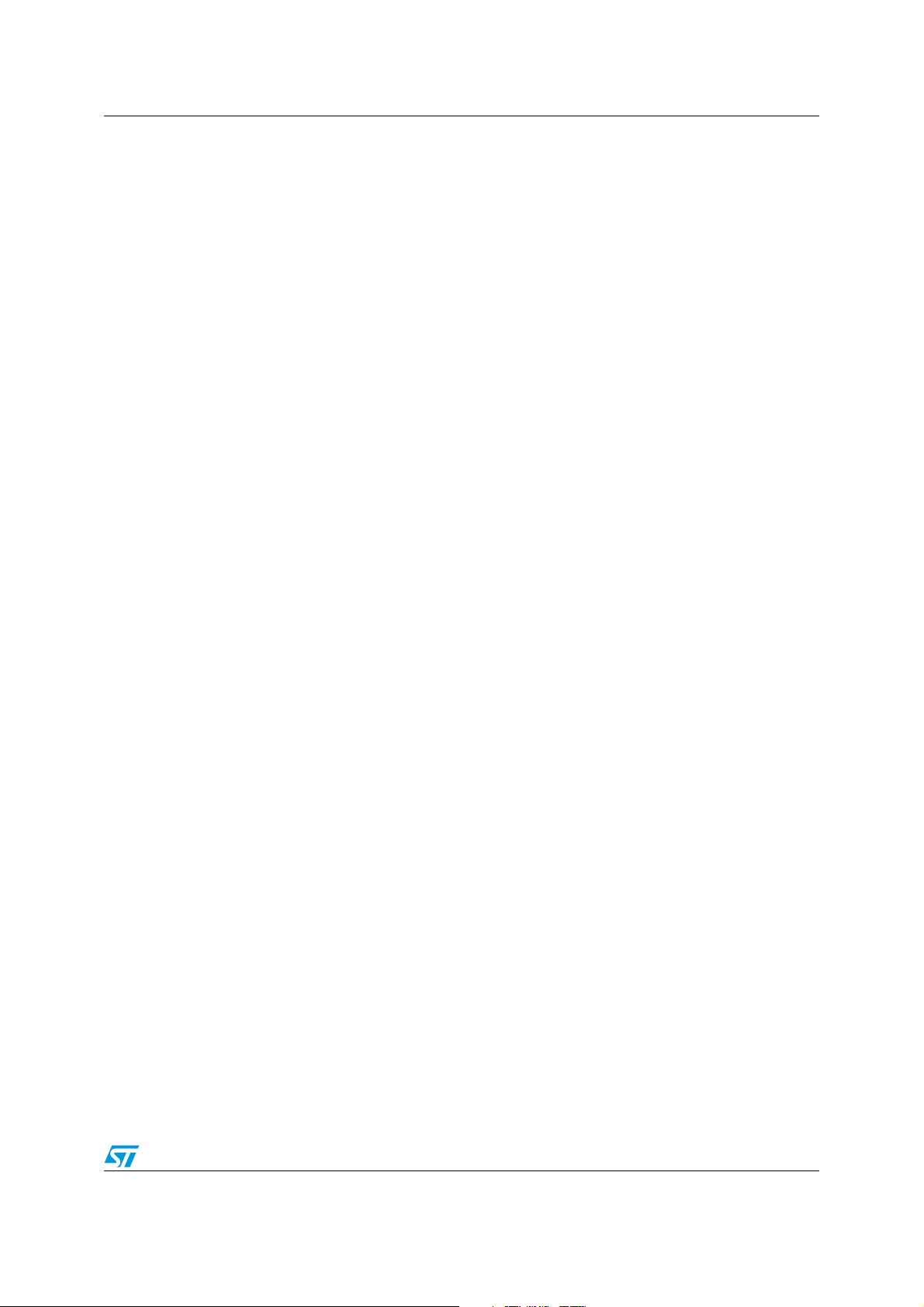
AN2557 List of tables
List of tables
Table 1. STM32F10xxx IAP implementation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 12
Table 2. Revision history . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 15
Doc ID 13588 Rev 8 3/16
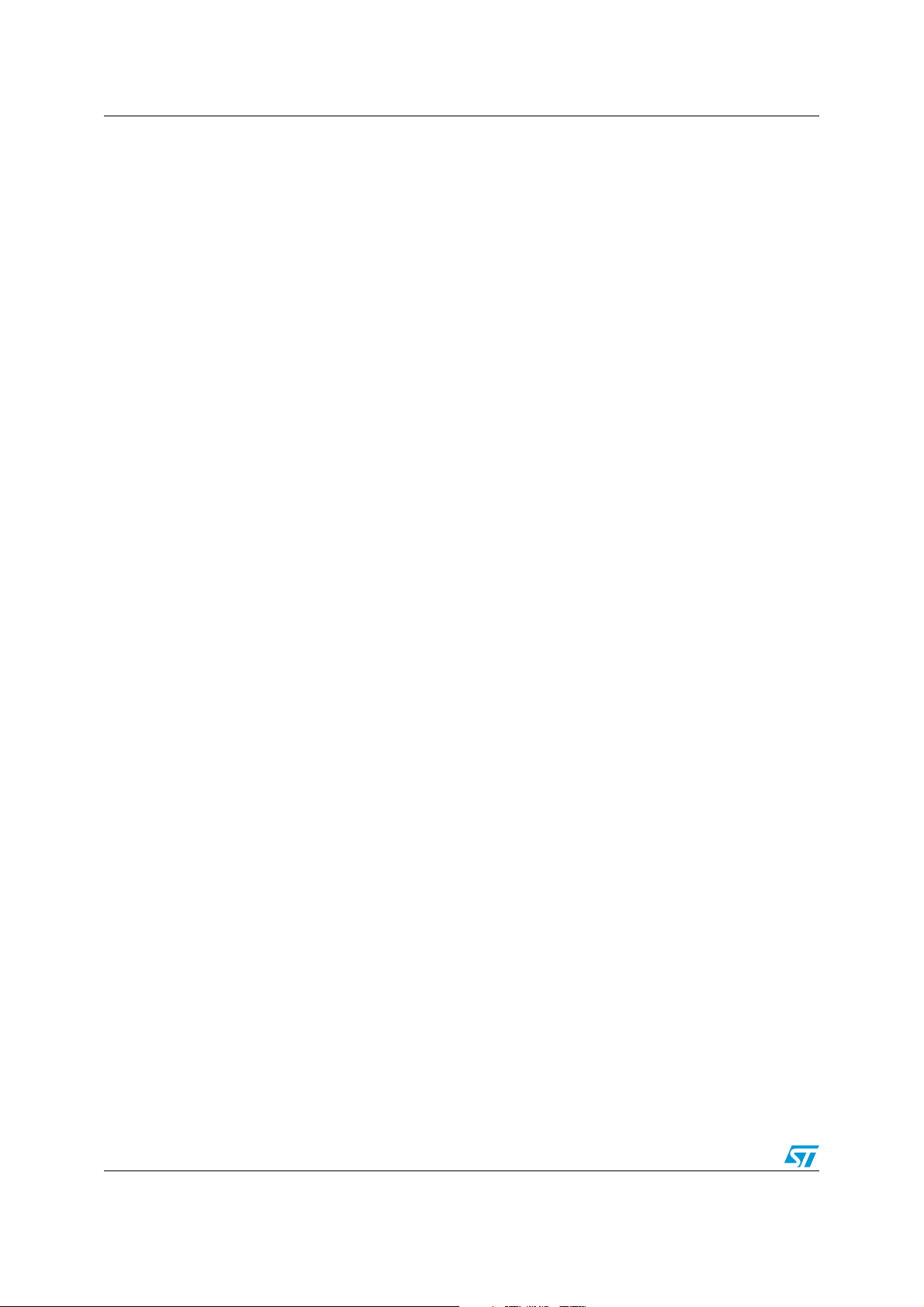
List of figures AN2557
List of figures
Figure 1. Flowchart of the IAP driver . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 7
Figure 2. COM port properties . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 8
Figure 3. IAP Driver menu when the STM32F10x Flash memory is not protected . . . . . . . . . . . . . . 10
Figure 4. IAP driver menu when the STM32F10x Flash memory is write-protected . . . . . . . . . . . . . 11
Figure 5. Flash memory usage . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14
4/16 Doc ID 13588 Rev 8
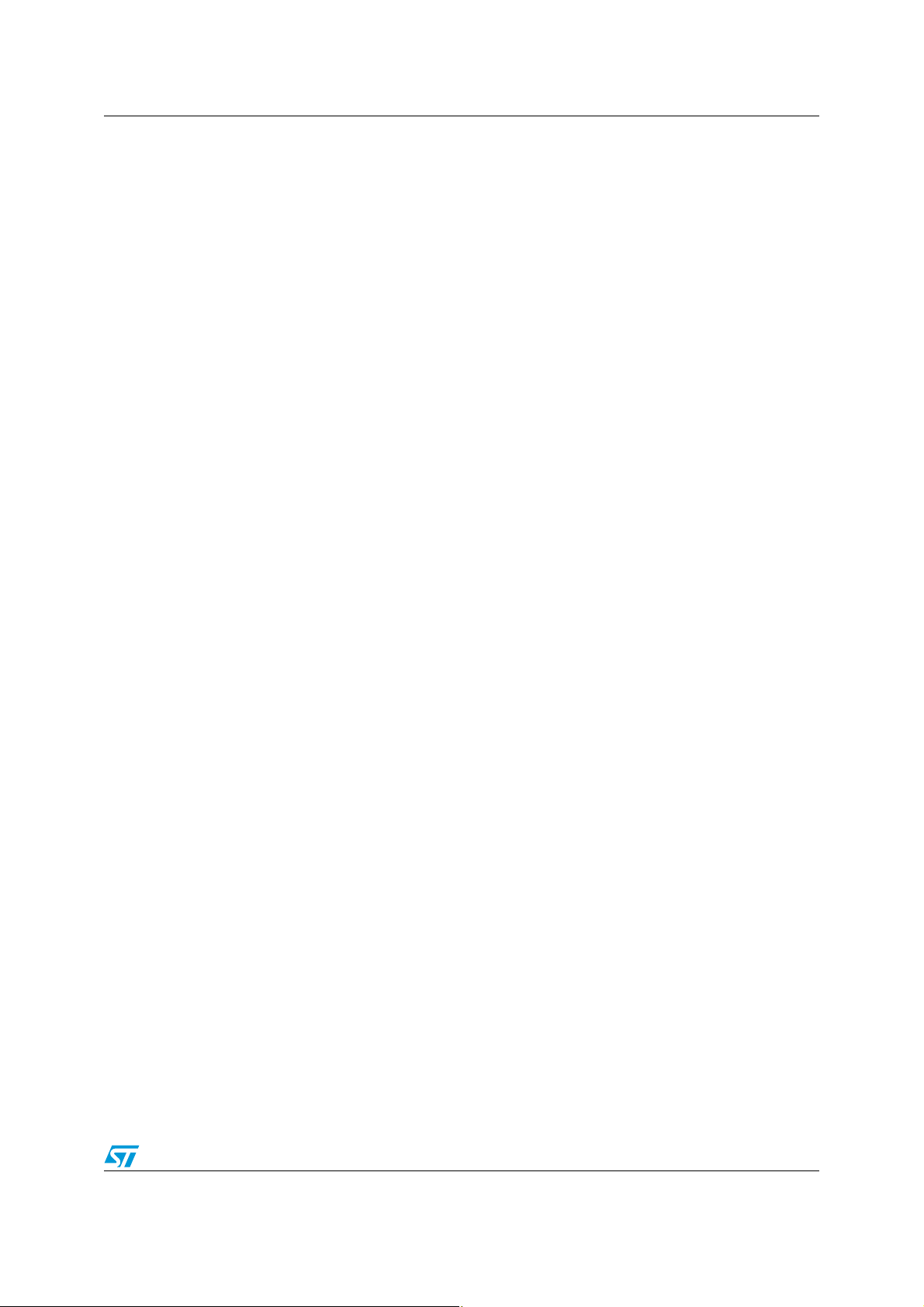
AN2557 IAP overview
1 IAP overview
Low-density devices are STM32F101xx, STM32F102xx, and STM32F103xx
microcontrollers where the Flash memory density ranges between 16 and 32 Kbytes.
Medium-density devices are STM32F101xx, STM32F102xx, and STM32F103xx
microcontrollers where the Flash memory density ranges between 64 and 128 Kbytes.
High-density devices are STM32F101xx and STM32F103xx microcontrollers where the
Flash memory density ranges between 256 and 512 Kbytes. High-density devices are
implemented in the STMicroelectronics STM3210E-EVAL evaluation board.
Connectivity line devices are STM32F105xx and STM32F107xx microcontrollers.
Connectivity line devices are implemented in the STMicroelectronics STM32100C-EVAL
evaluation board.
Low-density value line devices are STM32F100x4 and STM32F100x6 microcontrollers
where the Flash memory density ranges between 16 and 32 Kbytes.
Medium-density value line devices are STM32F100x8 and STM32F100xB
microcontrollers where the Flash memory density ranges between 64 and 128 Kbytes.
Medium-density value line devices are implemented in the STMicroelectronics STM32100BEVAL evaluation board.
XL-density devices are STM32F101xx and STM32F103xx microcontrollers where the
Flash memory density ranges between 768 Kbytes and 1 Mbyte.
High-density value line devices are STM32F100xC, STM32F100xD and STM32F100xE
microcontrollers where the Flash memory density ranges between 256 and 512 Kbytes.
High-density value line devices are implemented in the STMicroelectronics STM32100EEVAL evaluation board.
1.1 Principle
You should program the IAP driver to the Flash memory base address via the JTAG/SWD
interface using the development toolchain of your choice or the factory-embedded boot
loader in the System memory area.
'The IAP driver uses the USART to:
● Download a binary file from the HyperTerminal to the STM32F10x's internal Flash
memory.
● Upload the STM32F10x's internal flash memory content (starting from the defined user
application address) into a binary file.
● Execute the user program.
1.2 IAP driver description
The IAP driver contains the following set of source files:
● main.c: where the USART initialization is set. A main menu is then executed from the
common.c file.
● common.c: contains display functions and the main menu routine. The main menu
gives the options of loading a new binary file, uploading a new binary file, executing the
Doc ID 13588 Rev 8 5/16