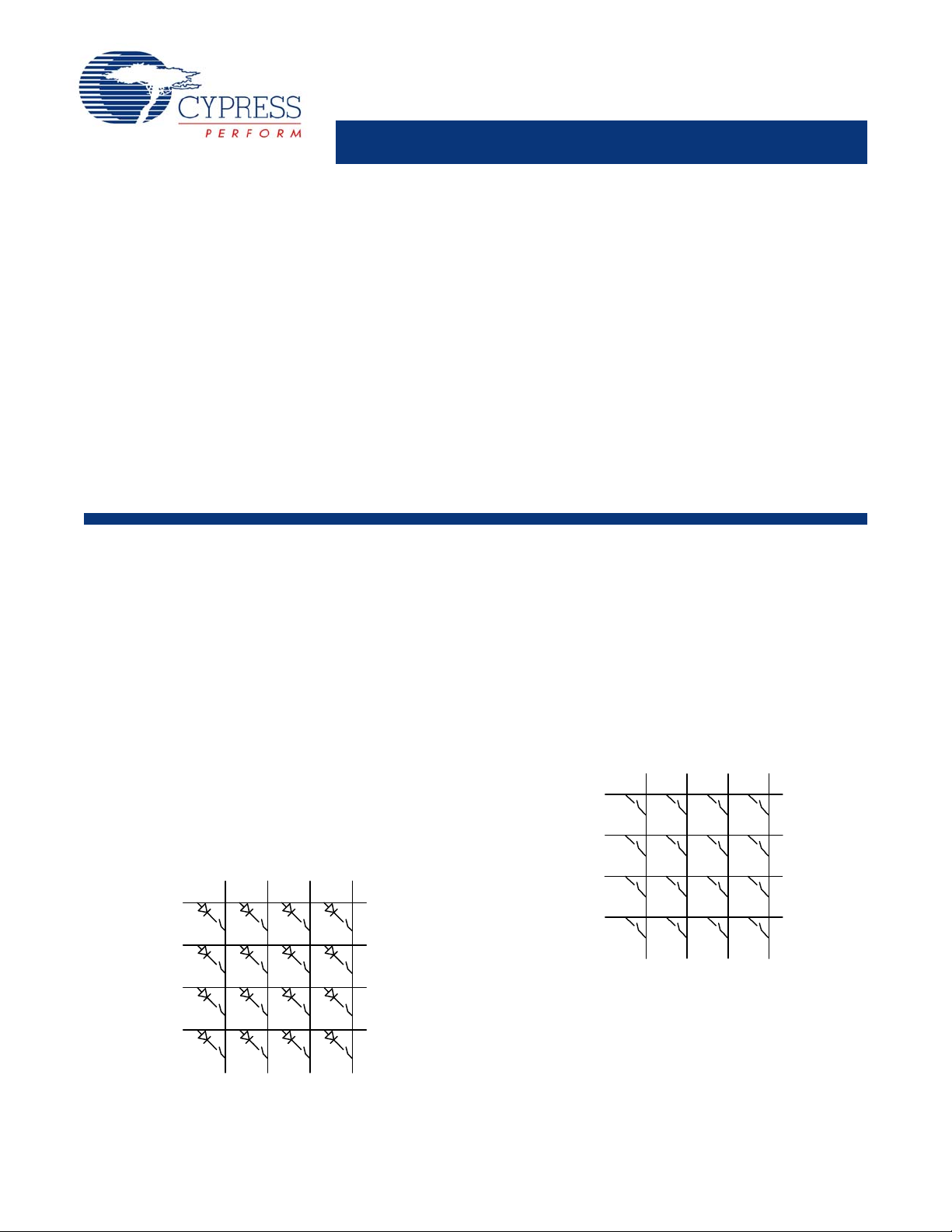
User Interface - Keypad Scan, PSoC® Style
AN2034
Author: Dave Van Ess
Associated Project: Yes
Associated Part Family: CY8C20x34, CY8C21x23, CY8C21x34
CY8C23x33, CY8C24x23A, CY8C24x94
CY8C27x43, CY8C29x66
GET FREE SAMPLES HERE
Software Version: PSoC Designer™ 5.0
Associated Application Notes: AN2354
Application Note Abstract
X–Y matrix keypads are an inexpensive interface enablin g interaction with microcontroller-based products. This application
note shows how the PSoC® microcontroller’s unique I/O structure can build a keypad scan routine that is fast, uses minimal
RAM resources, and operates in a polled or interrupt mode. A function c allable by either ‘C’ or assembly language is also
presented.
Introduction
An X-Y keypad enables use of N column lines and M r ow
lines to detect switch closures for N * M switches. For this
application note, a keypad is defined as an X-Y matrix
where only one key is pressed at a time, a s opposed to a
keyboard where simultaneous key closures are the norm
([Ctrl] [Shift] [Delete]). This keypad definition is valid for
telephones, calculators, security entry kiosks, or other
products where only one key is pressed at a time.
This application relies on PSoC General Purpose Input
Output (GPIO).
Rows and Columns
This application note uses C columns and R rows. Figure
1 shows an example of such a keypad:
Figure 1. 4-Column by 4-Row Keypad
C
C1C
0
R
0
[1,0]
[1,1]
[1,2]
[1,3]
[2,0]
[2,1]
[2,2]
[2,3]
[0,0]
R
1
[0,1]
R
2
[0,2]
R
3
[0,3]
2
[3,0]
[3,1]
[3,2]
[3,3]
C
3
Closure of switch [i, j] (column i, row j) enables current
flow from row j to column i. This keypad requires only eight
connections to the MCU. The 16 diodes can detect
multiple key closures. However, because PSoC
microcontroller reduces the cost of external components
such as op-amps, filters, and DACs, using 16 diodes is not
a good idea.
Well known techniques have been developed to detect
multiple key presses without diodes. Figure 2 shows the
keypad without diodes.
Figure 2. The Keypad you can Afford
C
C1C2C
0
R
0
[0,0]
[1,0]
[2,0]
R
1
[0,1]
[1,1]
[2,1]
R
2
[0,2]
[1,2]
[2,2]
R
3
[0,3]
[1,3]
[2,3]
3
[3,0]
[3,1]
[3,2]
[3,3]
The standard algorithm for reading a keypad is to
individually drive each row and sample the status of all
columns. Correctly combining all this information enables
detection of at least two simultaneous switch closures.
The hardware cost is less but there is software overhead
required to scan all four rows, read column status, and
condense this information into an answer.
To develop a keypad scan that is low in both hardware
and software resources, limit its operation to single-key
presses.
January 16, 2009 Document No. 001-40409 Rev. *A 1
[+] Feedback [+] Feedback
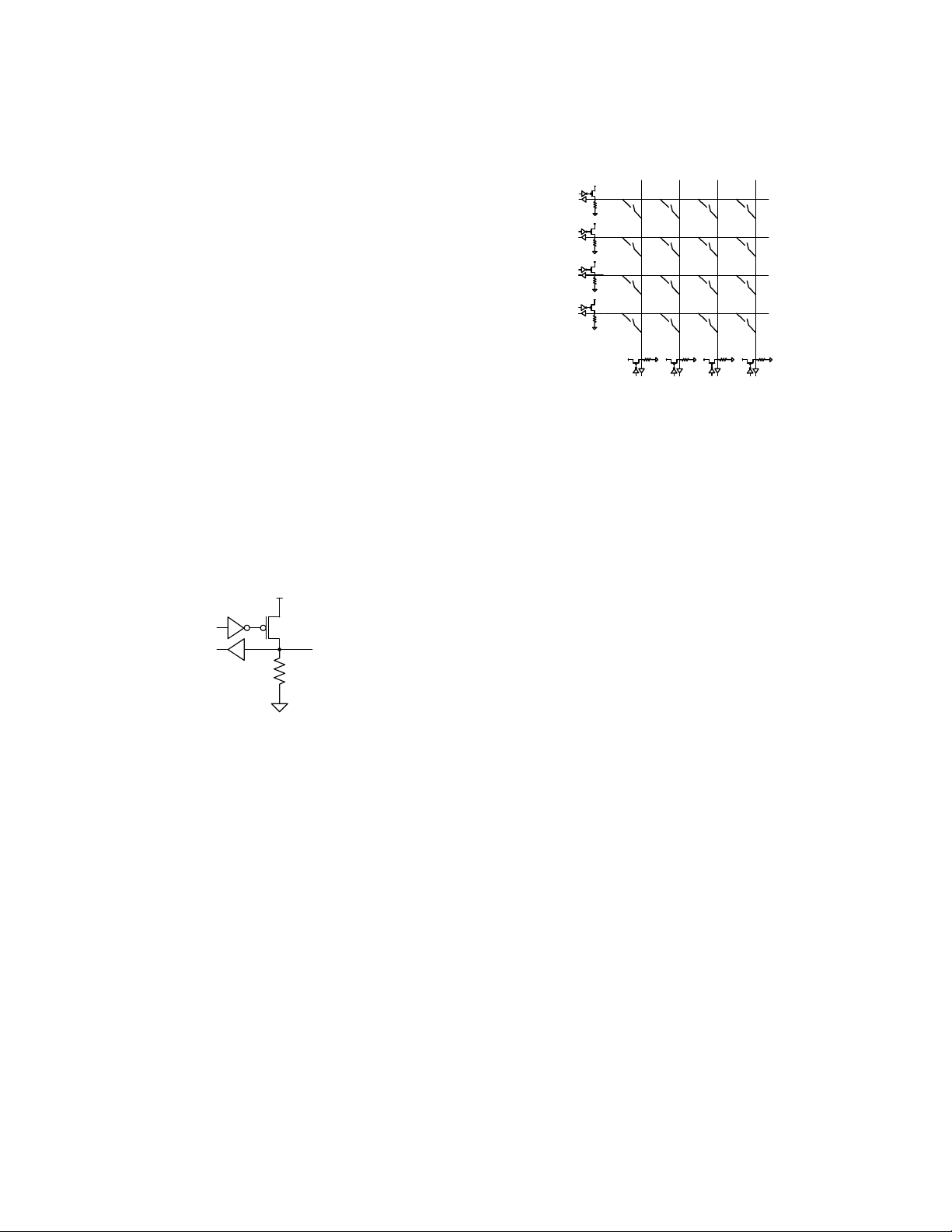
AN2034
PSOC General Purpose IO
To detect single-switch closures, use the following
algorithm:
Drive all rows simultaneously and read the columns.
Drive all columns simultaneously and read the rows.
Condense this data to determine switch-closure
status.
The structure of the general purpose of each pin simplifies
this bipolar use of rows and columns. Each pin has a
digital driver that can be set up to be:
Strong drive to V
Strong drive to V
and a pull down resistor to Vss.
DD
and a pull up resistor to VDD.
ss
High Impedance
Strong drive to either V
Use the pull down drive mode in the keypad scan routine,
as shown in Figure 3. Because it is also the default
condition for each pin at initial startup, there is no
requirement for special port configuration.
Figure 3. GPIO Set For Pull Down Mode
D
out
D
in
or Vss
DD
V
DD
pin
5.6 k
V
ss
Figure 4 shows implementation of a keypad scan.
Figure 4. PSoC Architecture for Keypad Scan
C0C1C2C
P
X.0
P
X.1
P
X.2
P
X.3
P
X.4PX.5PX.6PX.7
3
R
0
R
1
R
2
R
3
For this, press example switch [2,1] (column 2, row 1). The
algorithm reads the keypad in six steps.
Output b00001111 to the port. This drives all the
1.
rows high, leaving the columns passively pulled down.
2.
Read the port. The driven pins 0 through 3 remain
high and because the switch [2,1] is closed, pin 6 is
now high. The value read is b01001111.
3.
Output b11110000 to the port. This drives all the
columns high, leaving the rows passively pulled down.
4.
Read the port. The driven pins 4 through 7 remain
high and because the switch [2,1] is closed, pin 1 is
now high. The value read is b11110010.
5. “Anding” the result of step 2 and step 4 results in the
answer b01000010.
6. The upper 4 bits decode as column 2 and the lower 4
bits decode as row 1. This is a match with the closed
switch.
A subroutine that implements this algorithm is shown in
example Code 1. It enables the read ing of a four row by
four column keypad connected to port 1. It is found in
“
Keypad.asm,” located in the project file associated with
this application note. This subroutine uses eight
instructions, 15 bytes of program memory bytes, and 57CPU cycles.
January 16, 2009 Document No. 001-40409 Rev. *A 2
[+] Feedback [+] Feedback