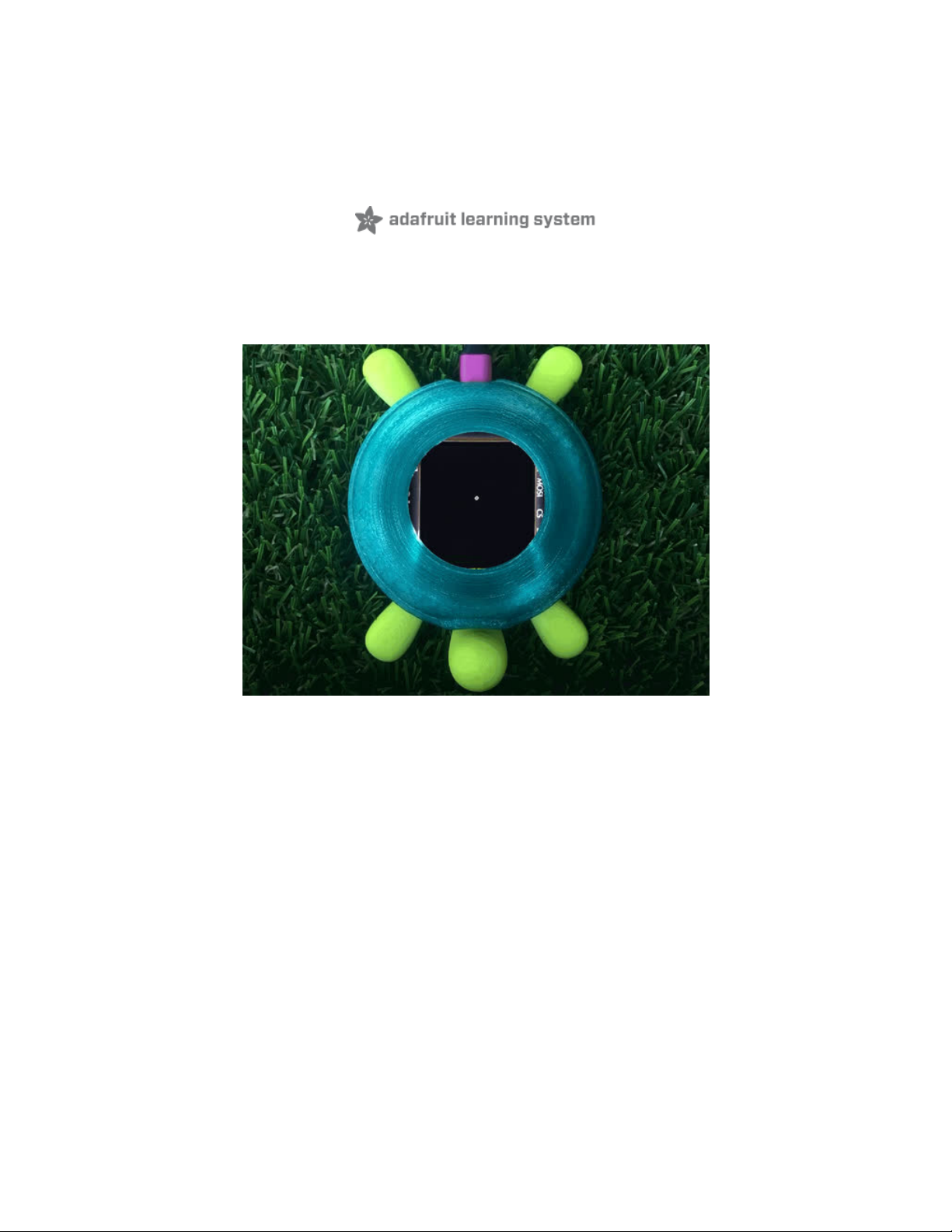
Turtle Graphics in CircuitPython on TFT Gizmo
Created by John Park
Last updated on 2021-02-08 06:46:25 PM EST
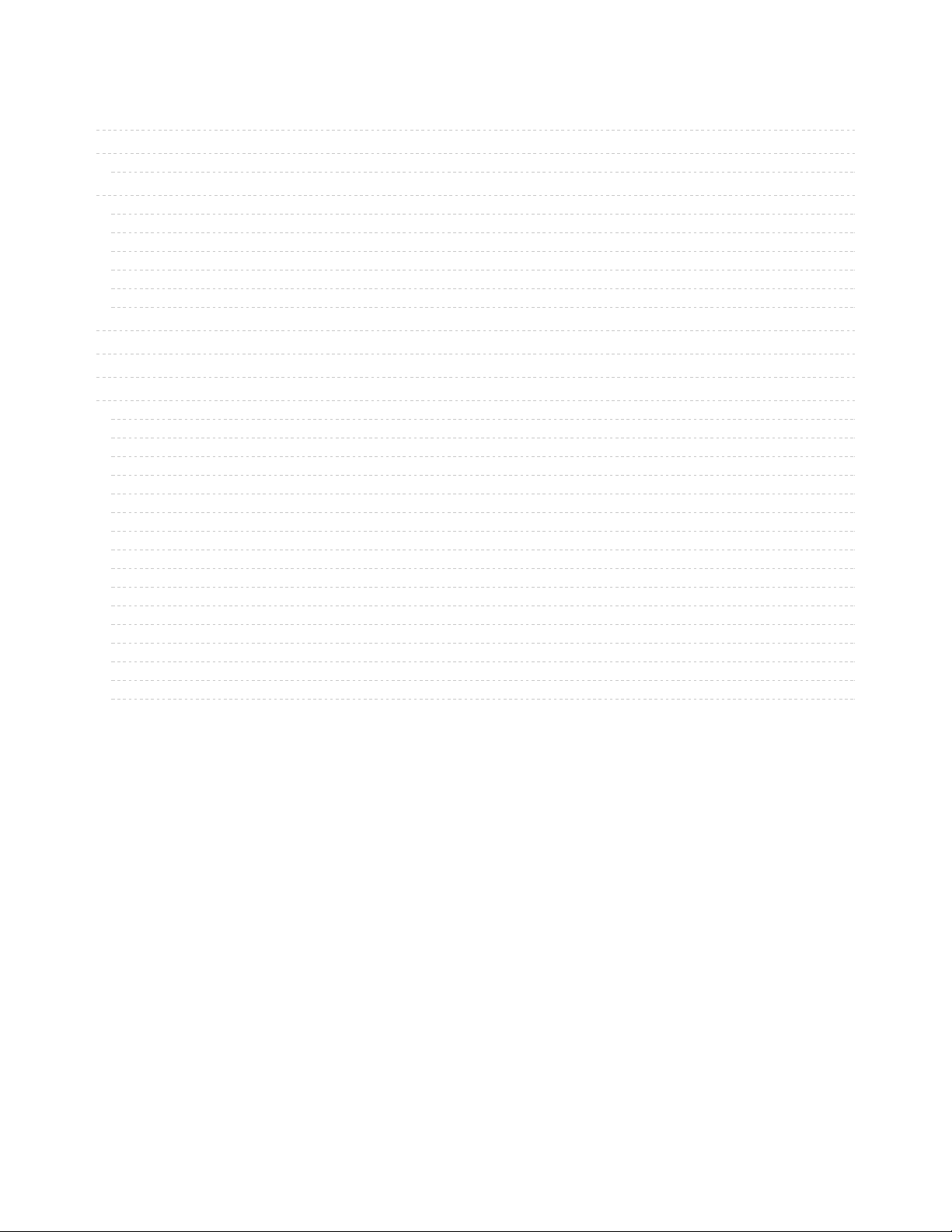
2
3
4
7
7
7
8
8
9
9
11
11
13
17
17
17
17
18
20
21
23
24
26
27
29
31
33
35
37
39
Guide Contents
Guide Contents
Overview
Parts
The Turtle API
Controlling the Pen
Moving
Heading
Shapes
More control
Queries
CircuitPython on Circuit Playground Bluefruit
Install or Update CircuitPython
Assembly
Turtle Graphics on Gizmo
Libraries
The Mu Editor
Turtle Graphics Code
Square
Asterisk
Circle
Circle Petals
Star
Rainbow Benzene
Parabolas
Sierpinski Triangle
Hilbert Curve
Koch Snowflake
Christmas Tree
Snowflakes
Where to Find More
© Adafruit Industries https://learn.adafruit.com/turtle-graphics-gizmo Page 2 of 40
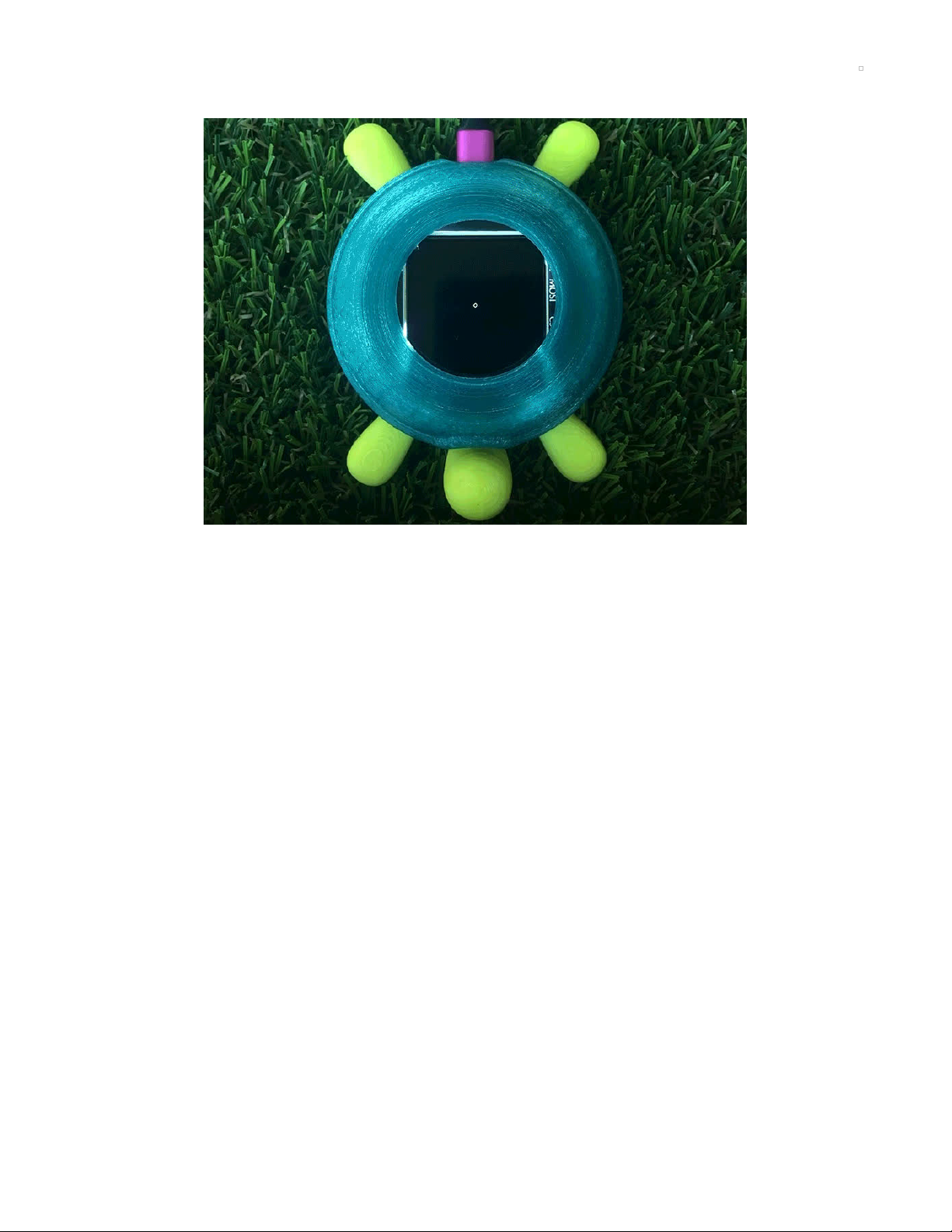
Overview
Learn to create your own graphics programs with Turtle Graphics on the Circuit Playground Bluefruit!
The legendary Turtle graphics of the Logo programming language has made their way to CircuitPython!
Now, you can learn to program your own graphics right on the TFT Gizmo screen attached to a Circuit
Playground Bluefruit board!
In this guide, we'll look at some general Turtle commands, then we'll set up the Circuit Playground
Bluefruit and TFT Gizmo to use Turtle in CircuitPython, and then we'll run some example programs to
draw sweet graphics on the display!
© Adafruit Industries https://learn.adafruit.com/turtle-graphics-gizmo Page 3 of 40
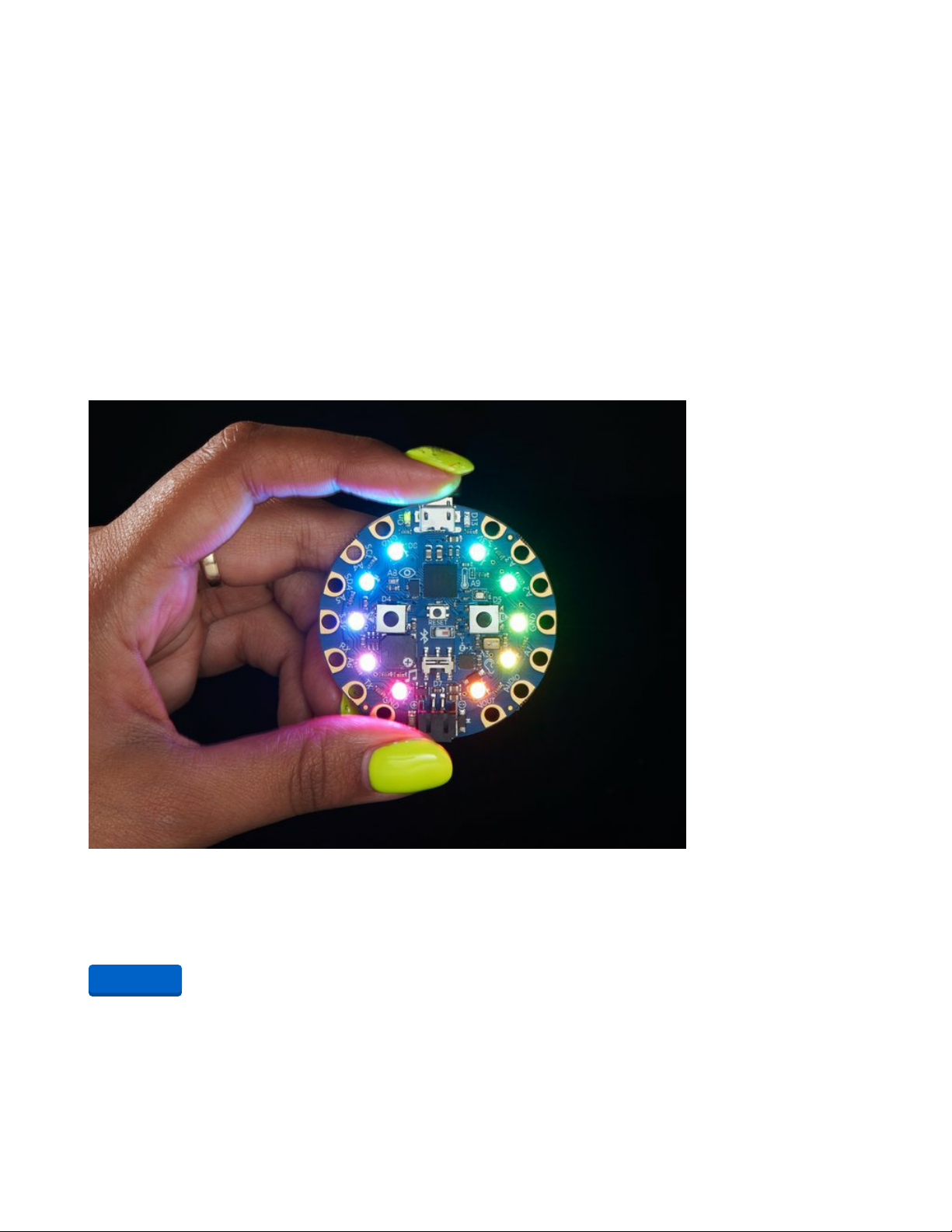
Besides the CPB with TFT Gizmo, the adafruit_turtle library will run on most of the M4 based boards,
such as the Hallowing M4, but will require some fine tuning of the pin assignments and other details.
You may have heard of, or even played around with,
turtle graphics
(https://adafru.it/FaL). Simply put, this
is a metaphor for drawing vector images where you control a
turtle
that can drag a pen along with it.
Commands to the turtle include things like move forward some distance, turn left or right by some angle,
lift the pen off the paper (so that moving won't make a mark), or put it on the paper (so that moving will
make a mark).
There have been many on-screen implementations of turtle graphics, most notably the
LOGO (https://adafru.it/FaM) programming language. Versions have also been made that control a robot
that has a pen so as to create on-paper copies of turtle drawings.
Parts
Circuit Playground Bluefruit - Bluetooth Low Energy
Circuit Playground Bluefruit is our third board in the Circuit Playground series, another step towards a
perfect introduction to electronics and programming. We've...
$24.95
In Stock
Your browser does not support the video tag.
Circuit Playground TFT Gizmo - Bolt-on Display + Audio Amplifier
Extend and expand your Circuit Playground projects with a bolt on TFT Gizmo that lets you add a lovely
color display in a sturdy and reliable fashion. This PCB looks just like a round...
$19.95
Add to Cart
© Adafruit Industries https://learn.adafruit.com/turtle-graphics-gizmo Page 4 of 40
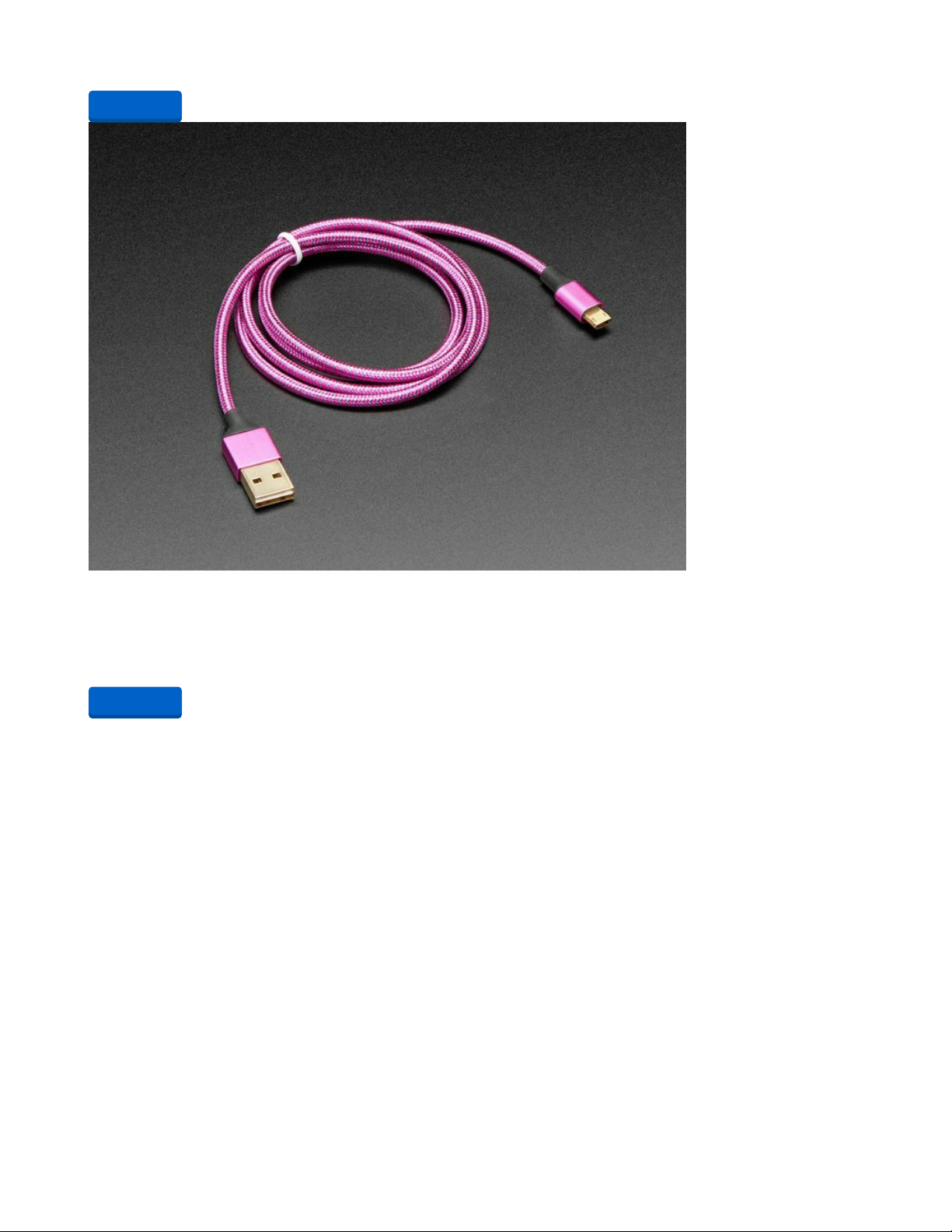
In Stock
Fully Reversible Pink/Purple USB A to micro B Cable - 1m long
This cable is not only super-fashionable, with a woven pink and purple Blinka-like pattern, it's also fully
reversible! That's right, you will save seconds a day by...
$3.95
In Stock
Add to Cart
Add to Cart
© Adafruit Industries https://learn.adafruit.com/turtle-graphics-gizmo Page 5 of 40
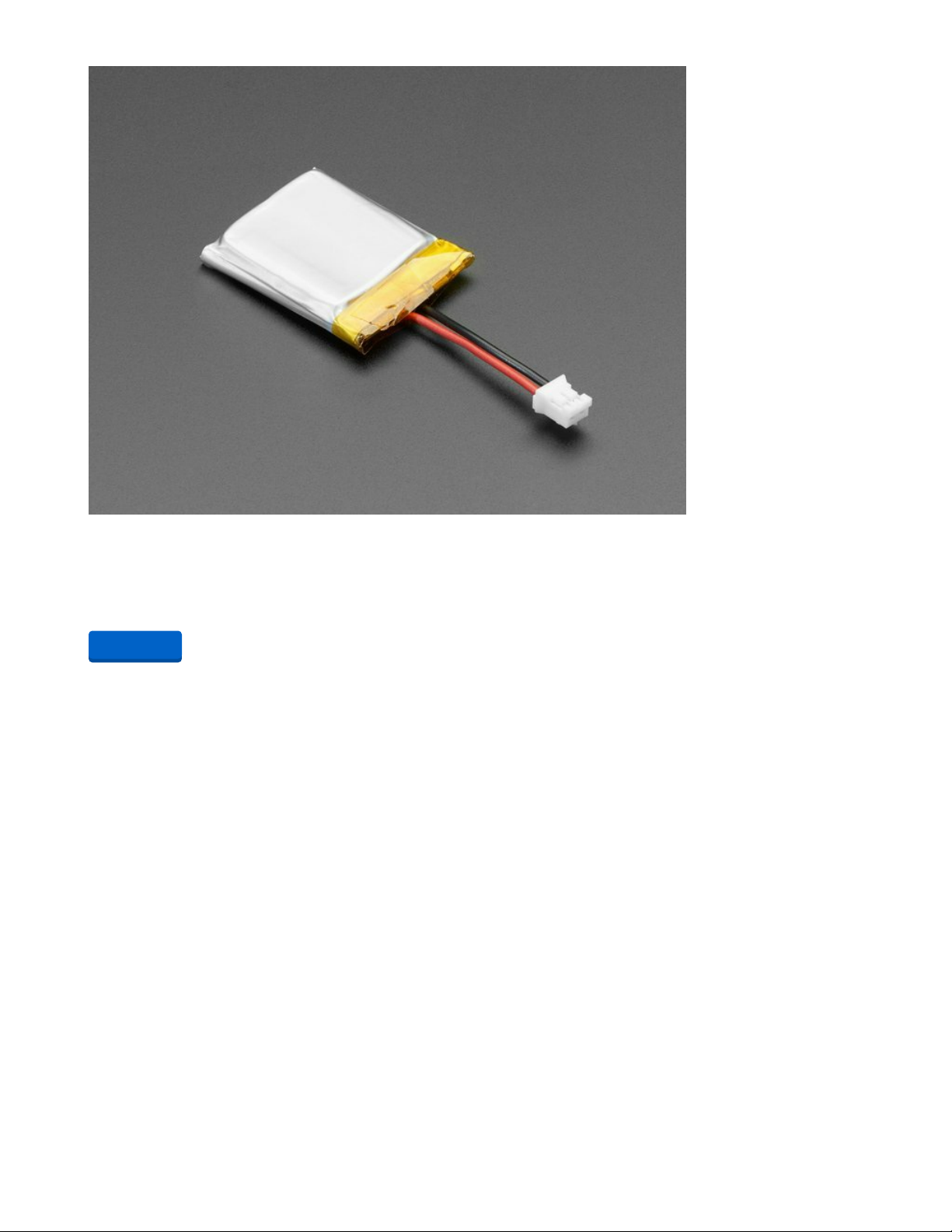
Lithium Ion Polymer Battery with Short Cable - 3.7V 350mAh
Lithium ion polymer (also known as 'lipo' or 'lipoly') batteries are thin, light and powerful. The output
ranges from 4.2V when completely charged to 3.7V. This battery...
$5.95
In Stock
Add to Cart
© Adafruit Industries https://learn.adafruit.com/turtle-graphics-gizmo Page 6 of 40
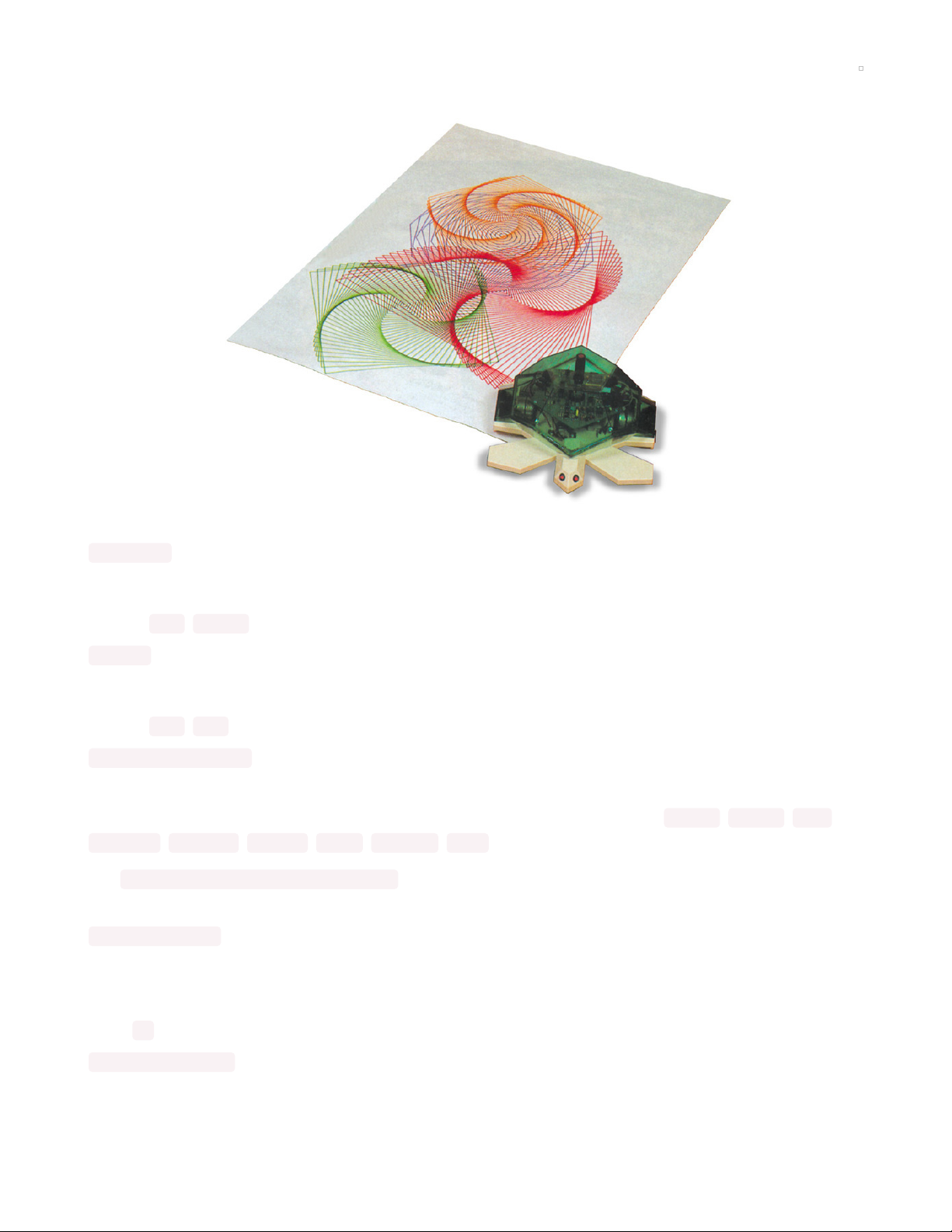
The Turtle API
Controlling the Pen
pendown()
Lower the pen, causing subsequent commands will draw.
Aliases: pd() , down()
penup()
Raise the pen, causing subsequent commands to not draw.
Alaises: pu() , up()
pencolor(color=None)
The form without an argument will return the current pen color as a 24-bit integer. The other form sets the
pen color to the specified value. The Color class should be used for this value: WHITE , BLACK , RED ,
ORANGE , YELLOW , GREEN , BLUE , PURPLE , PINK .
E.g. turtle.pencolor(adafruit_turtle.Color.RED)
Moving
forward(distance)
Move the turtle forward (on its current heading) by the specified distance. The distance is a number of
pixels if the turtle is moving exactly vertically or horizontally.
Alias: fd
backward(distance)
Move the turtle backward (opposite its current heading) by the specified distance. The distance is a
© Adafruit Industries https://learn.adafruit.com/turtle-graphics-gizmo Page 7 of 40
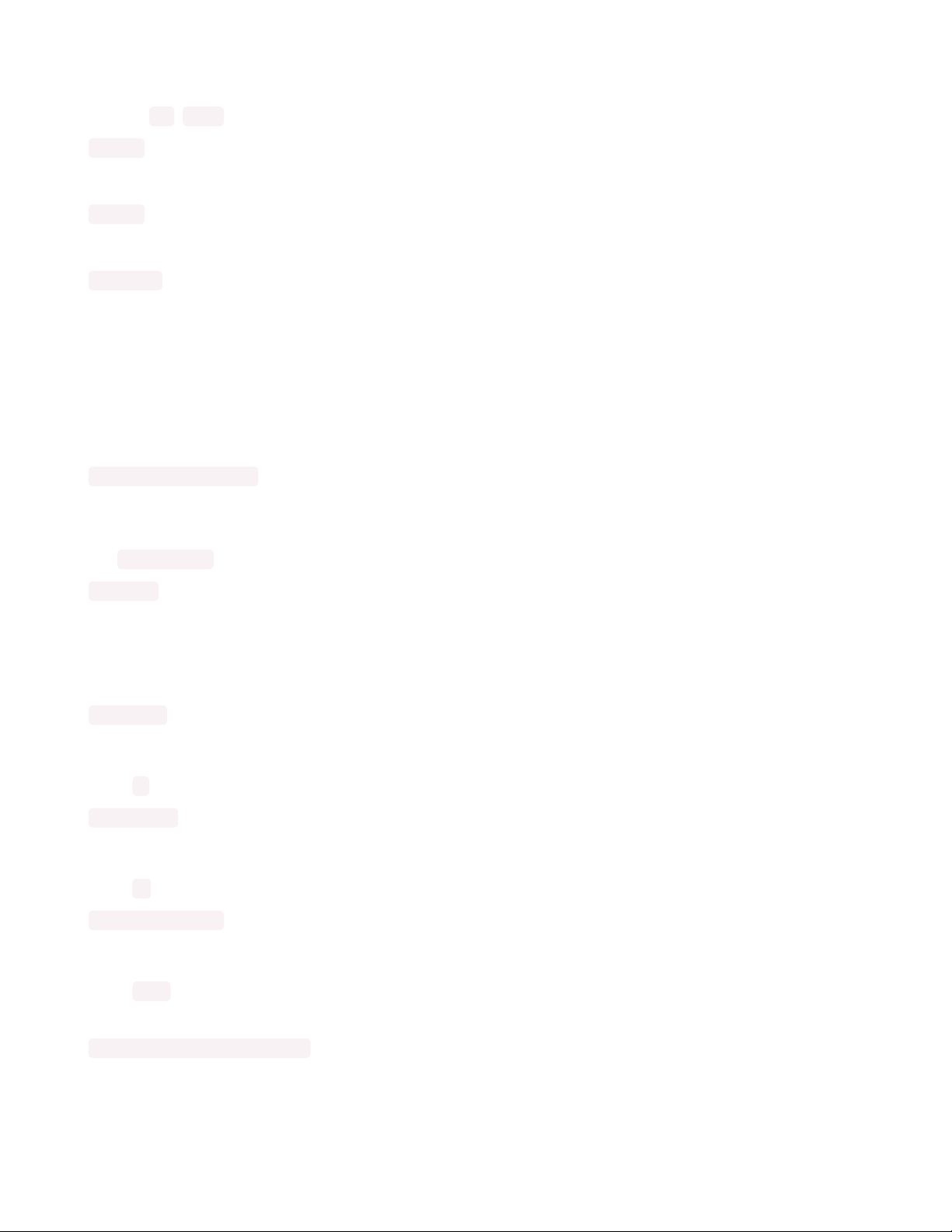
number of pixels if the turtle is moving exactly vertically or horizontally.
Aliases: bk , back
setx(x)
Set the turtle's horizontal coordinate.
sety(y)
Set the turtle's vertical coordinate.
goto(x, y)
Set both coordinates of the turtle.
Heading
Drawing in a straight line isn't that interesting, so the direction that the turtle is facing (and thus moving)
can be changed. This is called its
heading
. The first two functions below set what it means to change the
heading by some value. The result of calling these methods stay in effect until the next call to one of
them. By default, degrees are used, with a change of 1 corresponding to 1 degree.
degrees(fullcircle=360)
A full circle is 360 degrees and, by default, changing the heading by 1 means changing it by one degree.
Supplying a different value will serve to scale those incremental heading changes. For example, if you
call degrees(180) , changing the heading by 1 will change it by 2 degrees.
radians()
Use radians to turn the turtle. Changing the heading by 1 now means changing it by 1 radian (about 57.3
degrees).
The remaining methods change the turtle's heading.
left(angle)
Turn the turtle left by
angle
(what that means is subject to the above methods).
Alias: lt
right(angle)
Turn the turtle right by
angle
(what that means is subject to the above methods).
Alias: rt
setheading(angle)
Set the heading of the turtle to
angle
. Up is an
angle
of 0, right is 90.
Alias: seth
Shapes
dot(radius=None, color=None)
Draw a filled-in circle centered on the turtle's current position. Turtle position and heading are
unchanged.
© Adafruit Industries https://learn.adafruit.com/turtle-graphics-gizmo Page 8 of 40
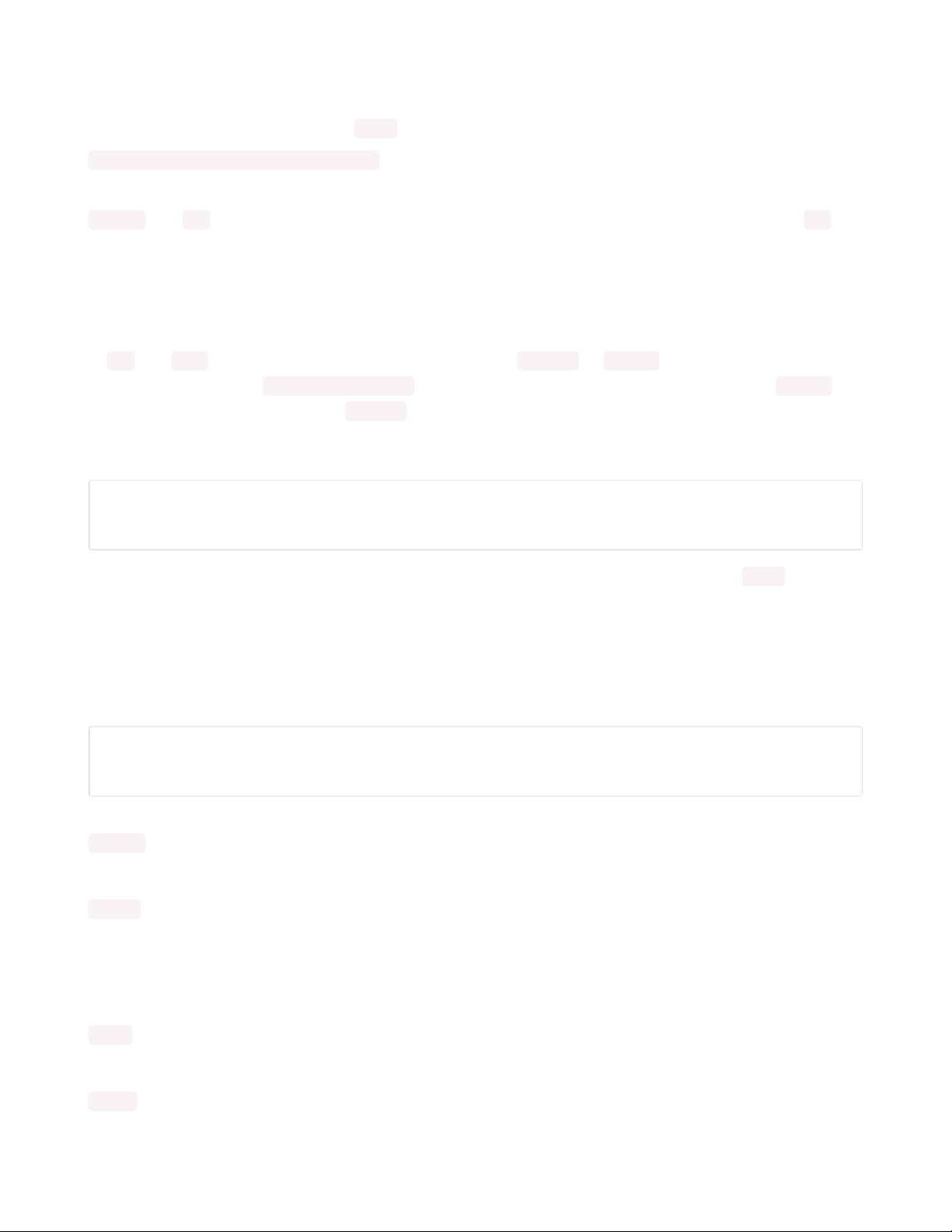
If
radius
is omitted a reasonable one is used, otherwise
radius
is used as the radius of the dot. If
color
is
omitted the current pen color is used, otherwise
color
is used. This does not change the pen's color. As
above, colors are available from the Color class.
circle(radius, extent=None, steps=None)
Draw a unfilled circle using the turtle's current pen color. This uses a computed sequence of calls to
forward and left so the turtle's position and heading are changed. Since the circle drawing uses left , it
draws counterclockwise by default. If
radius
is negative (i.e. < 0) drawing is done clockwise.
The radius of the desired circle is specified by
radius
, which is a number of pixels from the center to the
edge.
The argument
extent
specifies what portion of the circle to draw and is specified in the same units as calls
to left and right , as determined by the most recent call to degrees or radians . The default is to draw the
complete circle. Calling circle(10, extent=180) will draw half a circle of radius 10, assuming that radians
hasn't been called and neither has degrees with an argument other than 360. The turtle's heading is
always what it was after drawing the last part of the circle, regardless
extent
. This will always be at the
tangent to the circle. You can use this in your drawings. E.g. to draw a closed half-circle:
turtle.circle(20, extent=180)
turtle.left(90)
turtle.forward(40)
The final argument,
steps
, determines how many sides the circle has. By default (
steps
is None ) as many
are used as required to create a smooth circle. Specifying a value for
steps
has the effect of drawing that
many line segments instead. So circle(radius r, steps=6) will draw a hexagon.
If you draw a full circle, the turtle will end up back where it started with the heading it started with. By
drawing circles (of other polygons by using
steps
) repeatedly and turning between each, some
interesting designs can be created.
for _ in range(36):
turtle.circle(50, steps=6)
turtle.left(10)
More control
home()
Move the turtle to its initial position and heading.
clear()
Clear the screen, the position and heading of the turtle is unaffected.
Queries
These methods let you ask the turtle about aspects of it's current state.
pos()
Returns the turtle's (x, y) position.
xcor()
© Adafruit Industries https://learn.adafruit.com/turtle-graphics-gizmo Page 9 of 40
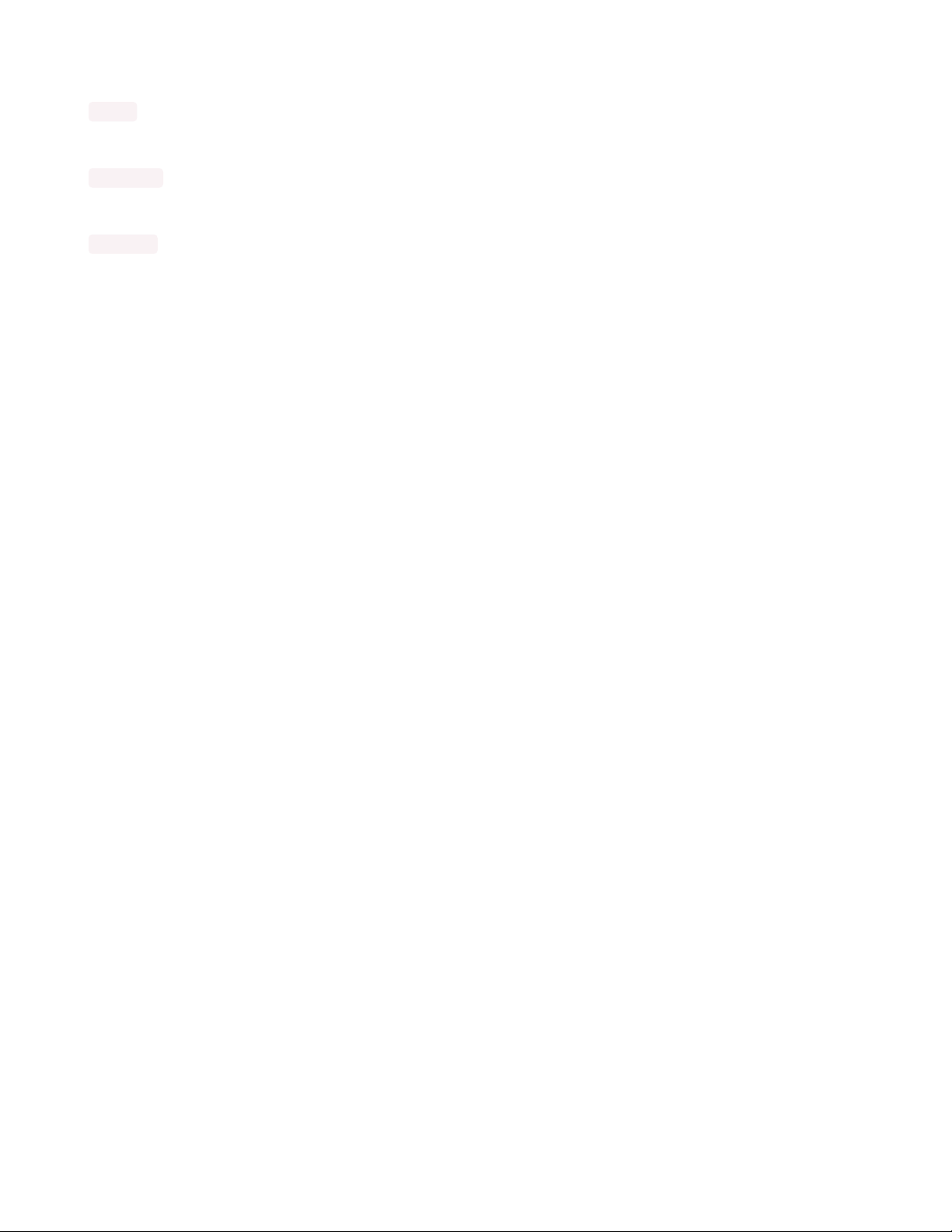
Returns the turtle's x coordinate.
ycor()
Returns the turtle's y coordinate.
heading()
Returns the turtle's heading.
isdown()
Returns whether the pen is down (i.e. drawing).
© Adafruit Industries https://learn.adafruit.com/turtle-graphics-gizmo Page 10 of 40
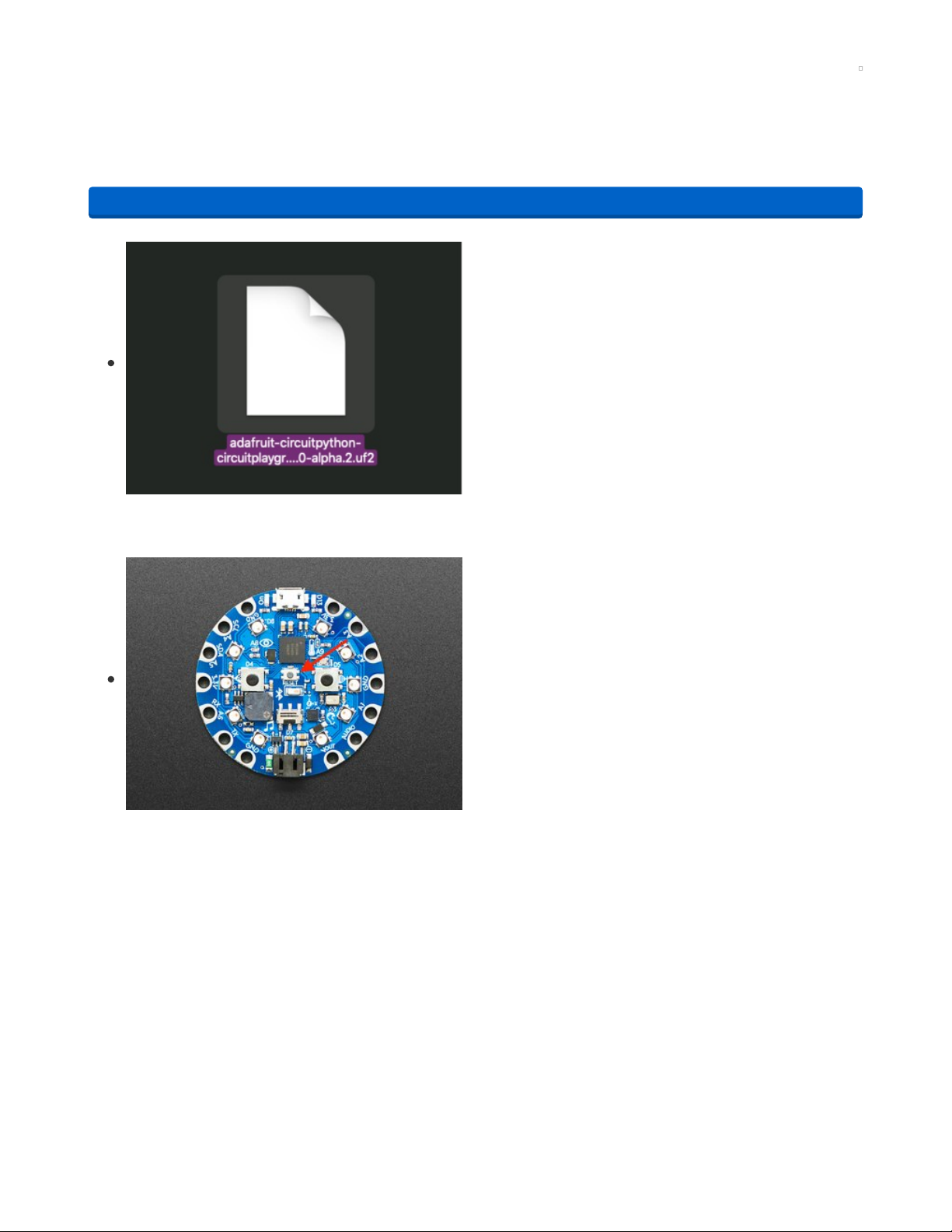
CircuitPython on Circuit Playground Bluefruit
Install or Update CircuitPython
Follow this quick step-by-step to install or update CircuitPython on your Circuit Playground Bluefruit.
https://adafru.it/FNK
Click the link above and download the latest UF2 file
Download and save it to your Desktop (or wherever is handy)
Plug your Circuit Playground Bluefruit into your computer
using a known-good data-capable USB cable.
A lot of people end up using charge-only USB cables and it
is very frustrating! So make sure you have a USB cable you
know is good for data sync.
Double-click the small Reset button in the middle of the CPB
(indicated by the red arrow in the image). The ten NeoPixel
LEDs will all turn red, and then will all turn green. If they turn
all red and stay red, check the USB cable, try another USB
port, etc. The little red LED next to the USB connector will
pulse red - this is ok!
If double-clicking doesn't work the first time, try again.
Sometimes it can take a few tries to get the rhythm right!
(If double-clicking doesn't do it, try a single-click!)
https://adafru.it/FNK
© Adafruit Industries https://learn.adafruit.com/turtle-graphics-gizmo Page 11 of 40
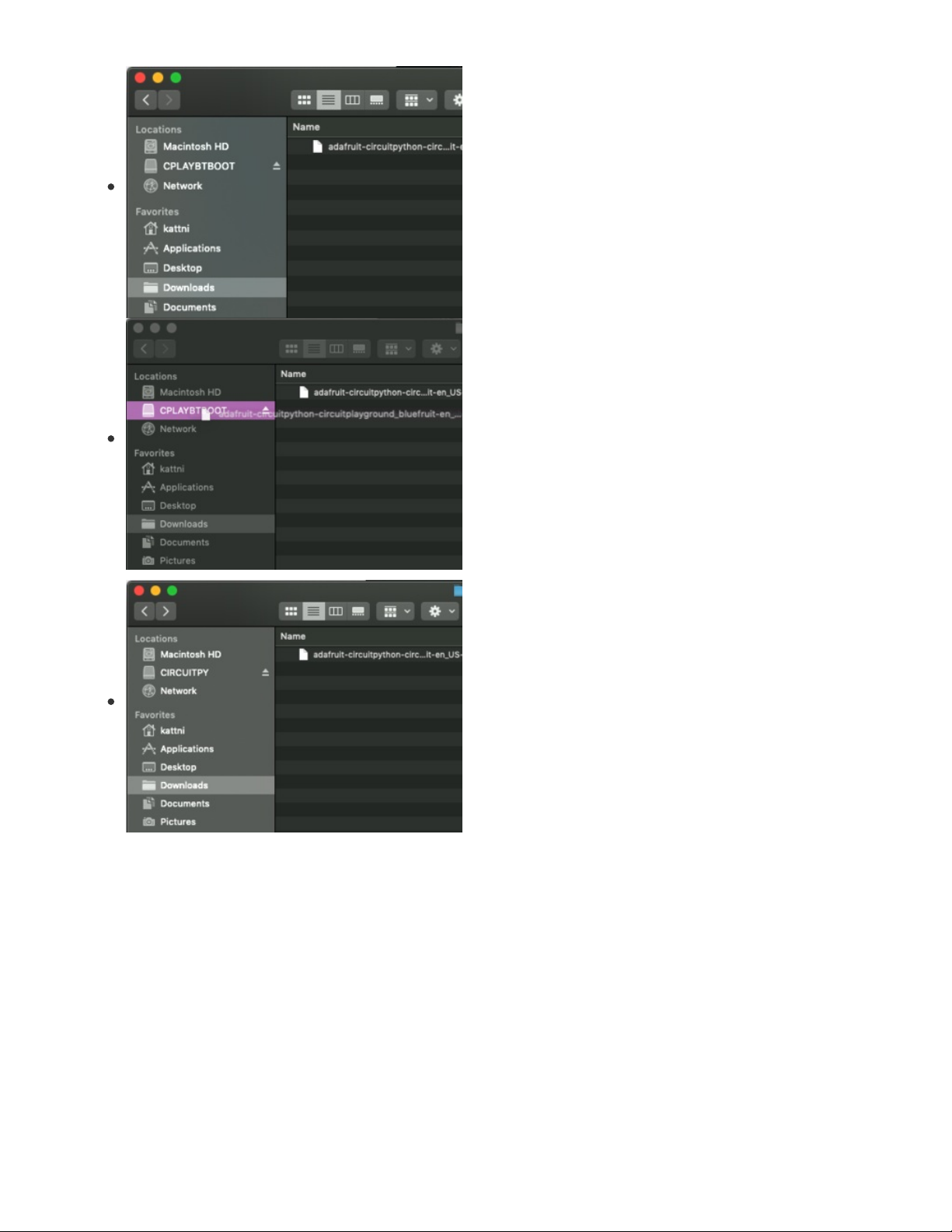
You will see a new disk drive appear called CPLAYBTBOOT.
Drag the adafruit_circuitpython_etc.uf2 file to
CPLAYBTBOOT.
The LEDs will turn red. Then, the CPLAYBTBOOT drive will
disappear and a new disk drive called CIRCUITPY will
appear.
That's it, you're done! :)
© Adafruit Industries https://learn.adafruit.com/turtle-graphics-gizmo Page 12 of 40